powershell 中使用长字符串 @
时间: 2024-10-28 10:12:52 浏览: 34
在PowerShell中,处理长字符串通常会遇到命令行界面字符限制的问题。当你需要输入较长的文本字符串时,可以使用反斜杠`\`对字符串进行分隔,并将其放在`@()`括号内,这是一种常见的工作-around技巧。这样做的原因是在 PowerShell 中,单独一行的字符串超过约8000个字符会被视为多行字符串,而使用 `@()` 可以将它们当作单个对象处理。
例如,如果你有一个非常长的字符串 `longStringHere`,你可以这样做:
```powershell
$longString = @"
longStringHere
This is a very long string that needs to be split over multiple lines.
It will be treated as one entity in PowerShell using the @() syntax.
@" | Out-String
# 现在变量$longString包含了整个长字符串,作为单个对象存储
```
通过这种方式,你可以安全地处理超出默认行宽的长字符串,并在后续操作中使用它。
相关问题
powershell脚本 文件查找字符串
PowerShell 提供了许多命令来搜索文件中的特定字符串,这通常涉及到文件内容的读取和过滤。其中最常用的是 `Get-Content` 和 `Where-Object` 或 `-match` 运算符。以下是基本步骤:
1. **使用 Get-Content -Path "C:\folder\*.*" | Where-Object { $_ -match "your_string" }
```
这里,`Get-Content` 将指定目录下的所有文件内容读取出来,`-match` 检查每一行是否包含指定的字符串。
2. **使用 Select-String**:
```powershell
Get-ChildItem C:\folder -File | Select-String -Pattern "your_string"
```
这将先获取文件夹内的所有文件,然后使用 `Select-String` 来筛选出包含指定字符串的那些。
3. **循环遍历文件**:
如果你想逐个文件检查,可以使用 `foreach` 循环:
```powershell
foreach ($file in (Get-ChildItem -Path "C:\folder\*.txt")) {
if (-not ([System.String]::IsNullOrEmpty($file.Content))) {
if ($file.Content -match "your_string") {
Write-Host "$file" contains the string.
}
}
}
```
这会逐个打开.txt文件,检查其内容是否包含目标字符串。
如何在PowerShell中替换特定文本字符串?
在PowerShell中,你可以使用`Replace-String`或`sed`(如果已经安装了Unix工具如Git Bash中的sed)来替换特定的文本字符串。这里是一个基本的例子:
如果你仅在纯PowerShell环境中操作,可以使用`[string]::Replace()`方法,它接受三个参数:源字符串、查找的模式和替换成的新值:
```powershell
$originalText = 'This is a sample text to be replaced.'
$newText = [System.Text.RegularExpressions.Regex]::Replace($originalText, 'sample', 'example')
Write-Host $newText
```
上述脚本会把字符串中的"sample"替换为"example"。
如果你想使用`sed`语法,如果你正在使用带有Unix工具的PowerShell(例如Git Bash),你可以这样做:
```powershell
# 首先,确保你的环境中有sed命令
$command = "sed -i 's/sample/example/g' yourFile.txt"
Invoke-Expression $command
```
这将在名为"yourFile.txt"的文件中全局替换所有的"sample"为"example"。
阅读全文
相关推荐
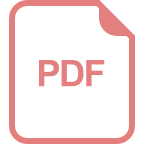
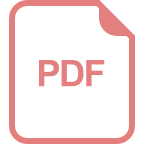
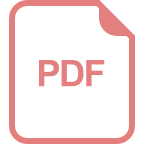













