flutter设计一个蓝牙控制页面,并写出代码,说明需要添加的依赖控件
时间: 2024-02-27 07:58:32 浏览: 109
好的,下面是Flutter设计蓝牙控制页面的代码。在此之前,需要安装flutter_blue插件来实现蓝牙的连接和控制。
```
import 'package:flutter/material.dart';
import 'package:flutter_blue/flutter_blue.dart';
class BluetoothControlPage extends StatefulWidget {
BluetoothControlPage({Key key}) : super(key: key);
@override
_BluetoothControlPageState createState() => _BluetoothControlPageState();
}
class _BluetoothControlPageState extends State<BluetoothControlPage> {
FlutterBlue flutterBlue = FlutterBlue.instance;
BluetoothDevice device;
BluetoothCharacteristic characteristic;
String _deviceName = '';
bool _isConnected = false;
bool _isStopped = true;
TextEditingController _textEditingController = TextEditingController();
@override
void initState() {
super.initState();
// 扫描蓝牙设备
flutterBlue.scanResults.listen((results) {
for (ScanResult result in results) {
if (result.device.name == 'YOUR_DEVICE_NAME') {
setState(() {
device = result.device;
_deviceName = device.name;
});
break;
}
}
});
// 开始扫描
flutterBlue.startScan(timeout: Duration(seconds: 4));
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('蓝牙控制'),
),
body: Container(
padding: EdgeInsets.all(16),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'设备名称:$_deviceName',
style: TextStyle(fontSize: 18),
),
SizedBox(height: 16),
RaisedButton(
child: Text(_isConnected ? '断开连接' : '连接设备'),
onPressed: _isConnected ? _disconnect : _connect,
),
SizedBox(height: 16),
TextFormField(
controller: _textEditingController,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: '发送内容',
),
),
SizedBox(height: 16),
RaisedButton(
child: Text(_isStopped ? '发送' : '停止发送'),
onPressed: _isConnected ? _sendData : null,
),
],
),
),
);
}
// 连接设备
void _connect() async {
if (device == null) return;
await device.connect();
List<BluetoothService> services = await device.discoverServices();
services.forEach((service) {
service.characteristics.forEach((characteristic) {
if (characteristic.uuid.toString() == 'YOUR_CHARACTERISTIC_UUID') {
setState(() {
this.characteristic = characteristic;
_isConnected = true;
});
}
});
});
}
// 断开连接
void _disconnect() async {
await device.disconnect();
setState(() {
_isConnected = false;
_isStopped = true;
});
}
// 发送数据
void _sendData() async {
if (_textEditingController.text.isEmpty) return;
if (_isStopped) {
setState(() {
_isStopped = false;
});
while (!_isStopped) {
await characteristic.write(_textEditingController.text.codeUnits);
await Future.delayed(Duration(milliseconds: 100));
}
} else {
setState(() {
_isStopped = true;
});
}
}
}
```
需要添加的依赖控件有:
- flutter_blue: 实现蓝牙连接和控制;
- TextFormField: 输入框控件。
阅读全文
相关推荐
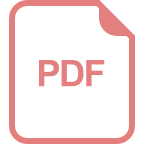
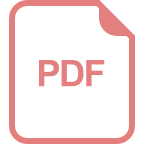
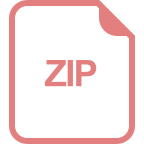
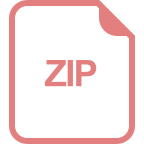
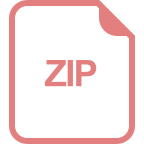
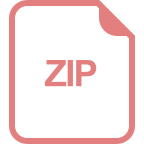
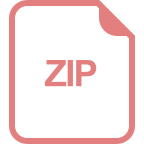
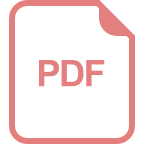
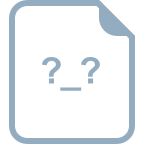
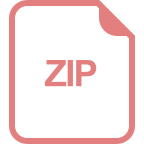
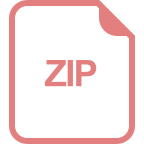
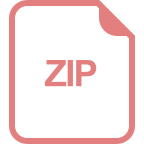
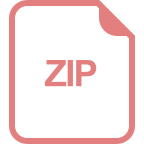
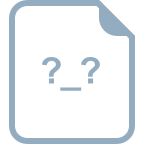