用python编写代码实现文本文件的文本可视化和分析
时间: 2023-09-17 14:08:47 浏览: 77
以下是一个使用Python实现文本文件文本可视化和分析的示例代码:
```python
import matplotlib.pyplot as plt
from wordcloud import WordCloud
import nltk
from nltk.corpus import stopwords
from collections import Counter
# 读取文本文件
with open('data.txt', 'r') as f:
text = f.read()
# 去除停用词
stop_words = set(stopwords.words('english'))
words = nltk.word_tokenize(text.lower())
words = [word for word in words if word.isalpha() and word not in stop_words]
# 统计词频
word_freq = Counter(words)
# 生成词云图
wordcloud = WordCloud(background_color='white').generate(text)
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
# 绘制词频统计图
labels, values = zip(*word_freq.items())
indexes = range(len(labels))
plt.bar(indexes, values)
plt.xticks(indexes, labels)
plt.show()
```
说明:
1. 首先使用`with open()`语句读取文本文件,将文本内容保存到变量`text`中。
2. 使用NLTK库中的`stopwords`语料库去除停用词,然后使用`nltk.word_tokenize()`函数将文本分词。
3. 使用Python标准库`collections`中的`Counter`类统计词频。
4. 使用`wordcloud`库生成词云图,并使用`matplotlib`库展示。
5. 使用`matplotlib`库绘制词频统计图。
可以根据具体需求对代码进行修改和扩展,例如增加情感分析和主题模型等功能。
相关推荐
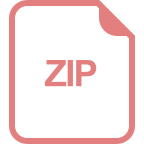
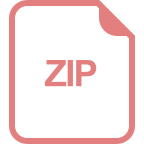
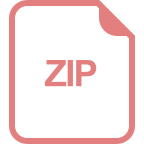
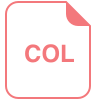
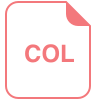
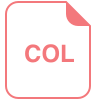
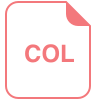










