上面那个问题中的数据可以对输出成一个json格式的文件么?
时间: 2024-02-20 17:57:20 浏览: 20
是的,可以将提取到的数据输出为 JSON 格式的文件。可以使用 C++ 中的第三方 JSON 库,例如 `nlohmann/json`。
以下是一个示例程序:
```c++
#include <iostream>
#include <fstream>
#include <regex>
#include <string>
#include <nlohmann/json.hpp>
using namespace std;
using json = nlohmann::json;
int main() {
ifstream infile("input.txt");
ofstream outfile("output.json");
if (!infile) {
cout << "Error opening input file" << endl;
return 1;
}
if (!outfile) {
cout << "Error opening output file" << endl;
return 1;
}
// 正则表达式匹配数字、英文字符串和中文
regex num_pattern("\\d+");
regex eng_pattern("[A-Za-z]+");
regex chi_pattern("[\\u4e00-\\u9fa5]+");
json output; // 存储输出数据的 JSON 对象
string line;
while (getline(infile, line)) {
json item; // 存储单个数据项的 JSON 对象
// 匹配数字
smatch num_match;
if (regex_search(line, num_match, num_pattern)) {
// 将匹配到的数字添加到数据项中
for (auto x : num_match) {
item["numbers"].push_back(x);
}
}
// 匹配英文字符串
smatch eng_match;
if (regex_search(line, eng_match, eng_pattern)) {
// 将匹配到的英文字符串添加到数据项中
for (auto x : eng_match) {
item["english"].push_back(x);
}
}
// 匹配中文
smatch chi_match;
if (regex_search(line, chi_match, chi_pattern)) {
// 将匹配到的中文添加到数据项中
for (auto x : chi_match) {
item["chinese"].push_back(x);
}
}
// 将单个数据项添加到输出对象中
output.push_back(item);
}
// 将输出对象写入 JSON 文件
outfile << output.dump(4);
infile.close();
outfile.close();
return 0;
}
```
这个程序会读取名为 "input.txt" 的文件,并将匹配到的数字、英文字符串和中文存储在一个 JSON 对象中,然后将该 JSON 对象输出到名为 "output.json" 的文件中。
相关推荐
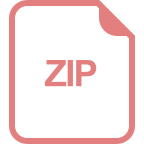
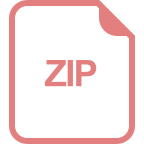
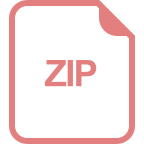














