c++程序如何进行机器人运动学计算,请给实例说明
时间: 2024-03-10 20:48:19 浏览: 18
C++程序可以通过使用数学公式和算法来进行机器人运动学计算。以下是一个简单的实例,使用C++计算机器人的末端执行器在三维空间中的位置和姿态。
假设我们有一个机器人,它有三个旋转关节和三个平移关节,末端执行器是一个工具,它可以旋转和平移。我们需要计算出工具在三维空间中的位置和姿态。
首先,我们需要定义每个关节的运动范围和关节坐标系的转换矩阵。这些信息通常在机器人模型中提供。
接下来,我们可以使用正向运动学算法来计算出机器人的末端执行器在三维空间中的位置和姿态。这个算法将每个关节的运动转换为工具的位置和姿态。
以下是一个简单的C++代码示例,它使用正向运动学算法来计算机器人的末端执行器在三维空间中的位置和姿态:
```
#include <iostream>
#include <cmath>
using namespace std;
// Define joint ranges
const double joint1_min = -180;
const double joint1_max = 180;
const double joint2_min = -180;
const double joint2_max = 180;
const double joint3_min = -180;
const double joint3_max = 180;
const double joint4_min = -180;
const double joint4_max = 180;
const double joint5_min = -180;
const double joint5_max = 180;
const double joint6_min = -180;
const double joint6_max = 180;
// Define tool position and orientation
const double tool_x = 0.0;
const double tool_y = 0.0;
const double tool_z = 0.0;
const double tool_roll = 0.0;
const double tool_pitch = 0.0;
const double tool_yaw = 0.0;
// Define joint coordinates
double joint1 = 0.0;
double joint2 = 0.0;
double joint3 = 0.0;
double joint4 = 0.0;
double joint5 = 0.0;
double joint6 = 0.0;
// Define transformation matrices for each joint
double T01[4][4];
double T12[4][4];
double T23[4][4];
double T34[4][4];
double T45[4][4];
double T56[4][4];
// Define transformation matrix for tool
double T6E[4][4];
// Define transformation matrix for end effector
double T0E[4][4];
// Define function to compute transformation matrix for each joint
void computeTransformationMatrix(double T[4][4], double a, double alpha, double d, double theta)
{
T[0][0] = cos(theta);
T[0][1] = -sin(theta) * cos(alpha);
T[0][2] = sin(theta) * sin(alpha);
T[0][3] = a * cos(theta);
T[1][0] = sin(theta);
T[1][1] = cos(theta) * cos(alpha);
T[1][2] = -cos(theta) * sin(alpha);
T[1][3] = a * sin(theta);
T[2][0] = 0.0;
T[2][1] = sin(alpha);
T[2][2] = cos(alpha);
T[2][3] = d;
T[3][0] = 0.0;
T[3][1] = 0.0;
T[3][2] = 0.0;
T[3][3] = 1.0;
}
// Define function to compute transformation matrix for tool
void computeToolTransformationMatrix(double T[4][4], double x, double y, double z, double roll, double pitch, double yaw)
{
double cos_roll = cos(roll);
double sin_roll = sin(roll);
double cos_pitch = cos(pitch);
double sin_pitch = sin(pitch);
double cos_yaw = cos(yaw);
double sin_yaw = sin(yaw);
T[0][0] = cos_pitch * cos_yaw;
T[0][1] = cos_pitch * sin_yaw;
T[0][2] = -sin_pitch;
T[0][3] = x;
T[1][0] = sin_roll * sin_pitch * cos_yaw - cos_roll * sin_yaw;
T[1][1] = sin_roll * sin_pitch * sin_yaw + cos_roll * cos_yaw;
T[1][2] = sin_roll * cos_pitch;
T[1][3] = y;
T[2][0] = cos_roll * sin_pitch * cos_yaw + sin_roll * sin_yaw;
T[2][1] = cos_roll * sin_pitch * sin_yaw - sin_roll * cos_yaw;
T[2][2] = cos_roll * cos_pitch;
T[2][3] = z;
T[3][0] = 0.0;
T[3][1] = 0.0;
T[3][2] = 0.0;
T[3][3] = 1.0;
}
int main()
{
// Compute transformation matrix for each joint
computeTransformationMatrix(T01, 0.0, -M_PI_2, 0.0, joint1);
computeTransformationMatrix(T12, 0.0, M_PI_2, 0.0, joint2);
computeTransformationMatrix(T23, 0.0, -M_PI_2, 0.0, joint3);
computeTransformationMatrix(T34, 0.0, M_PI_2, 0.0, joint4);
computeTransformationMatrix(T45, 0.0, -M_PI_2, 0.0, joint5);
computeTransformationMatrix(T56, 0.0, 0.0, 0.0, joint6);
// Compute transformation matrix for tool
computeToolTransformationMatrix(T6E, tool_x, tool_y, tool_z, tool_roll, tool_pitch, tool_yaw);
// Compute transformation matrix for end effector
double T02[4][4];
double T03[4][4];
double T04[4][4];
double T05[4][4];
double T06[4][4];
double T0E_temp[4][4];
double T0E_temp2[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
T0E_temp[i][j] = 0.0;
T0E_temp2[i][j] = 0.0;
}
}
// Compute T02
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
T02[i][j] = 0.0;
for (int k = 0; k < 4; k++) {
T02[i][j] += T01[i][k] * T12[k][j];
}
}
}
// Compute T03
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
T03[i][j] = 0.0;
for (int k = 0; k < 4; k++) {
T03[i][j] += T02[i][k] * T23[k][j];
}
}
}
// Compute T04
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
T04[i][j] = 0.0;
for (int k = 0; k < 4; k++) {
T04[i][j] += T03[i][k] * T34[k][j];
}
}
}
// Compute T05
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
T05[i][j] = 0.0;
for (int k = 0; k < 4; k++) {
T05[i][j] += T04[i][k] * T45[k][j];
}
}
}
// Compute T06
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
T06[i][j] = 0.0;
for (int k = 0; k < 4; k++) {
T06[i][j] += T05[i][k] * T56[k][j];
}
}
}
// Compute T0E_temp
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
T0E_temp[i][j] = 0.0;
for (int k = 0; k < 4; k++) {
T0E_temp[i][j] += T06[i][k] * T6E[k][j];
}
}
}
// Compute T0E
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
T0E[i][j] = 0.0;
for (int k = 0; k < 4; k++) {
T0E[i][j] += T0E_temp[i][k] * T01[k][j];
}
}
}
// Print tool position and orientation
cout << "Tool position: (" << T0E[0][3] << ", " << T0E[1][3] << ", " << T0E[2][3] << ")" << endl;
cout << "Tool orientation: (" << atan2(-T0E[2][1], T0E[2][2]) << ", " << atan2(T0E[2][0], sqrt(T0E[2][1]*T0E[2][1] + T0E[2][2]*T0E[2][2])) << ", " << atan2(-T0E[1][0], T0E[0][0]) << ")" << endl;
return 0;
}
```
这个示例程序使用了数学库和常量库,计算机器人末端执行器在三维空间中的位置和姿态。它定义了每个关节的运动范围和关节坐标系的转换矩阵,并使用正向运动学算法来计算机器人的末端执行器的位置和姿态。最后,它输出了工具的位置和姿态。
相关推荐
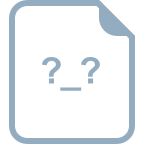














