用python语言写一个小游戏,用WASD分别控制角色:小人的上下左右移动,小人前方随机掉落障碍物,小人不能成功躲避障碍物,则游戏结束
时间: 2023-05-22 16:04:03 浏览: 125
好的,这个小游戏可以使用Python的Pygame库来实现,以下是一个参考代码:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 游戏界面尺寸
WIDTH = 500
HEIGHT = 500
# 颜色定义
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
# 创建游戏界面
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("小游戏")
# 加载图像
person_img = pygame.image.load("person.png")
person_rect = person_img.get_rect()
obstacle_img = pygame.image.load("obstacle.png")
obstacle_rect = obstacle_img.get_rect()
# 初始化游戏状态
person_x = WIDTH // 2
person_y = HEIGHT // 2
obstacle_x = random.randint(0, WIDTH - obstacle_rect.width)
obstacle_y = -obstacle_rect.height
score = 0
running = True
# 游戏主循环
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 清空屏幕
screen.fill(WHITE)
# 更新障碍物位置
obstacle_y += 5
if obstacle_y > HEIGHT:
obstacle_x = random.randint(0, WIDTH - obstacle_rect.width)
obstacle_y = -obstacle_rect.height
score += 1
# 绘制障碍物
screen.blit(obstacle_img, (obstacle_x, obstacle_y))
# 绘制小人
screen.blit(person_img, (person_x, person_y))
# 检测碰撞
if person_rect.colliderect(obstacle_rect.move(obstacle_x, obstacle_y)):
running = False
# 更新小人位置
key = pygame.key.get_pressed()
if key[pygame.K_w]:
person_y -= 5
if key[pygame.K_s]:
person_y += 5
if key[pygame.K_a]:
person_x -= 5
if key[pygame.K_d]:
person_x += 5
# 边界限制
person_x = max(0, min(person_x, WIDTH - person_rect.width))
person_y = max(0, min(person_y, HEIGHT - person_rect.height))
# 显示得分
font = pygame.font.Font(None, 24)
text = font.render("得分: {}".format(score), True, BLACK)
screen.blit(text, (10, 10))
# 刷新屏幕
pygame.display.flip()
# 退出Pygame
pygame.quit()
```
注意,这个代码中使用了两个用到的图像,一个是小人的图像(`person.png`),一个是障碍物的图像(`obstacle.png`)。你可以在合适的位置放置这两张图片文件。
当游戏运行时,你可以使用 WASD 键来控制小人的移动。随机生成的障碍物会从屏幕顶部往下落,如果小人没有成功躲避障碍物,游戏就会结束。最后,屏幕上方会显示当前得分。
相关推荐
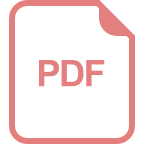














