c++ opencv 光流
时间: 2023-06-30 11:27:10 浏览: 58
光流是计算相邻两帧图像中像素点的运动信息的一种方法。在 OpenCV 中,可以使用 calcOpticalFlowPyrLK 函数来计算光流。以下是一个简单的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main() {
VideoCapture cap("test.mp4");
if (!cap.isOpened()) {
cout << "Failed to open video" << endl;
return -1;
}
Mat old_frame, old_gray;
vector<Point2f> old_points;
// 读取第一帧图像并提取角点
cap.read(old_frame);
cvtColor(old_frame, old_gray, COLOR_BGR2GRAY);
goodFeaturesToTrack(old_gray, old_points, 100, 0.3, 7, Mat(), 7, false, 0.04);
// 创建窗口
namedWindow("Optical Flow", WINDOW_NORMAL);
while (true) {
Mat frame, gray;
vector<Point2f> new_points;
vector<uchar> status;
vector<float> err;
// 读取当前帧图像并转为灰度图像
cap.read(frame);
if (frame.empty()) {
break;
}
cvtColor(frame, gray, COLOR_BGR2GRAY);
// 计算光流
calcOpticalFlowPyrLK(old_gray, gray, old_points, new_points, status, err);
// 绘制光流
for (int i = 0; i < old_points.size(); i++) {
if (status[i]) {
Point2f pt1 = old_points[i];
Point2f pt2 = new_points[i];
line(frame, pt1, pt2, Scalar(0, 255, 0), 2);
circle(frame, pt2, 5, Scalar(0, 0, 255), -1);
}
}
// 显示结果
imshow("Optical Flow", frame);
if (waitKey(30) == 27) {
break;
}
// 更新先前帧的信息
old_gray = gray.clone();
old_points = new_points;
}
cap.release();
destroyAllWindows();
return 0;
}
```
在上面的代码中,首先读取第一帧图像并使用 goodFeaturesToTrack 函数提取角点。然后,循环读取每一帧图像,计算光流并绘制结果。最后更新先前帧的信息。在计算光流时,可以使用 calcOpticalFlowFarneback 函数实现更高级的光流计算。
相关推荐
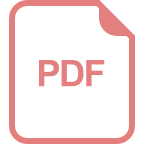














