试写一个名为shmysql的类,主要功能是,从shmysql.json中读取mysql数据库连接信息,利用连接信息建立一个连接池,该类支持上下文管理,能够通过with shmysql() as cs 返回一个游标操作数据库
时间: 2024-09-26 09:10:45 浏览: 35
当然,这里是一个简单的Python类设计,名为`ShMysql`,它实现了从`shmysql.json`中读取数据库连接信息,并构建一个连接池。同时,它符合上下文管理协议,可以方便地在`with`语句中使用:
```python
import json
import pymysql.cursors
from contextlib import contextmanager
class ShMysql:
def __init__(self):
try:
with open('shmysql.json', 'r', encoding='utf-8') as f:
config = json.load(f)
except FileNotFoundError:
print("shmysql.json not found, please make sure it exists.")
return
self.config = config
self.pool = None
@property
def connection(self):
if self.pool is None:
self.pool = self.create_connection_pool()
return self.pool
@staticmethod
def create_connection_pool(config):
db_params = {
'user': config['user'],
'password': config['password'],
'host': config['host'],
'port': config['port'],
'db': config['database'],
'cursorclass': pymysql.cursors.DictCursor # 使用DictCursor以便于结果以字典形式返回
}
return pymysql.Pool(**db_params)
@contextmanager
def get_db_cursor(self):
conn = self.connection
cursor = conn.cursor()
try:
yield cursor
finally:
cursor.close()
conn.close()
def __enter__(self):
return self.get_db_cursor()
def __exit__(self, exc_type, exc_val, exc_tb):
pass # 如果需要处理异常,这里添加相应代码
# 使用示例
if __name__ == "__main__":
with ShMysql() as cs:
query = "SELECT * FROM table_name"
cs.execute(query)
results = cs.fetchall()
for result in results:
print(result)
```
在这个类中,`get_db_cursor`方法是上下文管理器的核心,当使用`with shmysql() as cs`时,会自动在进入和退出时关闭游标和连接。注意,实际应用中可能需要处理异常情况。
阅读全文
相关推荐
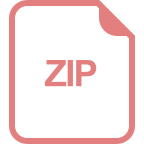
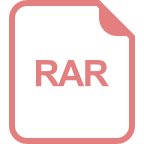
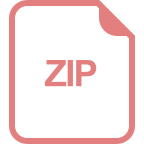
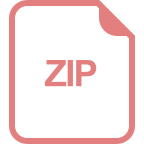
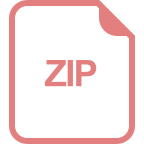
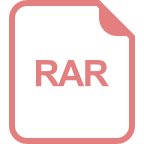
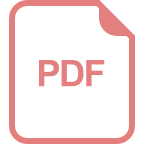
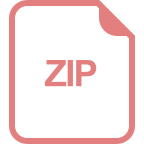
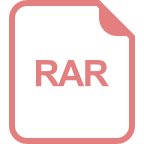
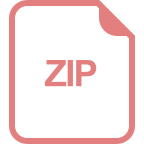
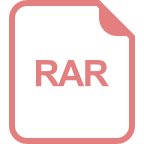
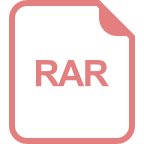
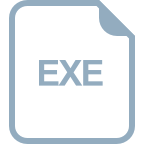
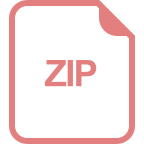
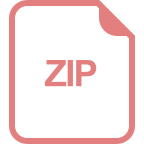
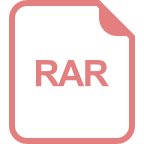
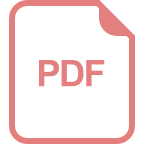
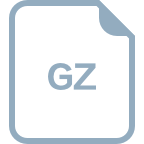