写一个登录页面,登录后显示本地EXCEL数据
时间: 2024-03-24 21:37:18 浏览: 17
好的,我可以提供一个简单的登录页面的示例代码,前端使用HTML、CSS和JavaScript,后端使用Node.js和Express框架,读取本地Excel数据使用了xlsx库。
前端代码(login.html):
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login Page</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="login-box">
<h2>Login Here</h2>
<form>
<div class="user-box">
<input type="text" name="username" required="">
<label>Username</label>
</div>
<div class="user-box">
<input type="password" name="password" required="">
<label>Password</label>
</div>
<button type="submit" class="btn">Login</button>
</form>
</div>
<script src="login.js"></script>
</body>
</html>
```
CSS代码(style.css):
```css
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: sans-serif;
}
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
background: #6c7ae0;
}
.login-box {
background: #fff;
padding: 40px;
border-radius: 10px;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.2);
text-align: center;
}
.login-box h2 {
margin-bottom: 30px;
color: #333;
}
.user-box {
position: relative;
}
.user-box input {
width: 100%;
padding: 10px 0;
margin-bottom: 30px;
border: none;
border-bottom: 2px solid #333;
outline: none;
background: none;
font-size: 16px;
color: #333;
}
.user-box label {
position: absolute;
top: 0;
left: 0;
padding: 10px 0;
font-size: 16px;
color: #333;
pointer-events: none;
transition: 0.5s;
}
.user-box input:focus ~ label,
.user-box input:valid ~ label {
top: -20px;
left: 0;
color: #6c7ae0;
font-size: 12px;
}
.btn {
display: inline-block;
background: #6c7ae0;
color: #fff;
padding: 10px 30px;
margin-top: 40px;
border: none;
border-radius: 30px;
cursor: pointer;
transition: 0.5s;
}
.btn:hover {
transform: translateY(-5px);
box-shadow: 0 5px 20px rgba(0, 0, 0, 0.2);
}
```
JavaScript代码(login.js):
```javascript
const form = document.querySelector("form");
form.addEventListener("submit", async (event) => {
event.preventDefault();
const username = form.elements.username.value;
const password = form.elements.password.value;
const response = await fetch(`/login?username=${username}&password=${password}`);
const data = await response.json();
if (data.success) {
renderTable(data.excelData);
} else {
alert(data.message);
}
});
function renderTable(excelData) {
const table = document.createElement("table");
const thead = document.createElement("thead");
const tbody = document.createElement("tbody");
const headerRow = document.createElement("tr");
excelData.header.forEach((header) => {
const th = document.createElement("th");
th.textContent = header;
headerRow.appendChild(th);
});
thead.appendChild(headerRow);
excelData.rows.forEach((row) => {
const tr = document.createElement("tr");
row.forEach((cell) => {
const td = document.createElement("td");
td.textContent = cell;
tr.appendChild(td);
});
tbody.appendChild(tr);
});
table.appendChild(thead);
table.appendChild(tbody);
document.body.innerHTML = "";
document.body.appendChild(table);
}
```
后端代码(server.js):
```javascript
const express = require("express");
const xlsx = require("xlsx");
const app = express();
const PORT = 3000;
app.use(express.static("public"));
app.get("/login", (req, res) => {
const username = req.query.username;
const password = req.query.password;
if (username === "admin" && password === "admin") {
const workbook = xlsx.readFile("data.xlsx");
const sheetName = workbook.SheetNames[0];
const worksheet = workbook.Sheets[sheetName];
const excelData = xlsx.utils.sheet_to_json(worksheet, { header: 1 });
res.json({ success: true, excelData: { header: excelData[0], rows: excelData.slice(1) } });
} else {
res.json({ success: false, message: "Invalid username or password" });
}
});
app.listen(PORT, () => {
console.log(`Server started on port ${PORT}`);
});
```
在本地创建一个名为"data.xlsx"的Excel文件,填入一些数据,然后将上面的代码保存到相应的文件中,运行命令`npm install express xlsx`安装依赖包,然后运行命令`node server.js`启动服务器,打开浏览器,访问`http://localhost:3000/login.html`,输入用户名和密码(admin/admin),点击登录按钮,就可以看到本地Excel数据被渲染成了一个HTML表格。
相关推荐
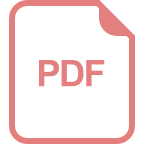
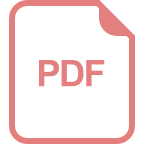
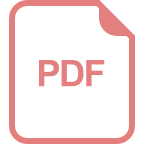
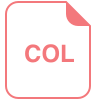
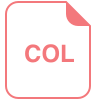
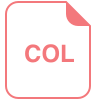
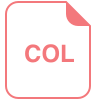
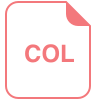









