用C语言实现这些操作
时间: 2024-02-23 17:02:15 浏览: 16
下面是用C语言实现循环队列的基本操作的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 10 // 定义循环队列的最大长度
typedef struct {
int *data; // 存储队列元素的数组指针
int front; // 队头指针
int rear; // 队尾指针
} CircularQueue;
// 初始化循环队列
void initQueue(CircularQueue *queue) {
queue->data = (int*)malloc(sizeof(int) * MAX_SIZE);
queue->front = 0;
queue->rear = 0;
}
// 判断队列是否为空
int isEmpty(CircularQueue *queue) {
return queue->front == queue->rear;
}
// 判断队列是否已满
int isFull(CircularQueue *queue) {
return (queue->rear + 1) % MAX_SIZE == queue->front;
}
// 入队操作
void enqueue(CircularQueue *queue, int value) {
if (isFull(queue)) {
printf("Queue is full!\n");
return;
}
queue->data[queue->rear] = value;
queue->rear = (queue->rear + 1) % MAX_SIZE;
}
// 出队操作
int dequeue(CircularQueue *queue) {
if (isEmpty(queue)) {
printf("Queue is empty!\n");
return -1;
}
int value = queue->data[queue->front];
queue->front = (queue->front + 1) % MAX_SIZE;
return value;
}
// 获取队头元素
int getFront(CircularQueue *queue) {
if (isEmpty(queue)) {
printf("Queue is empty!\n");
return -1;
}
return queue->data[queue->front];
}
// 获取队列长度
int getSize(CircularQueue *queue) {
return (queue->rear - queue->front + MAX_SIZE) % MAX_SIZE;
}
// 清空队列
void clearQueue(CircularQueue *queue) {
queue->front = 0;
queue->rear = 0;
}
int main() {
CircularQueue queue;
initQueue(&queue);
enqueue(&queue, 1);
enqueue(&queue, 2);
enqueue(&queue, 3);
printf("Queue size: %d\n", getSize(&queue));
printf("Queue front: %d\n", getFront(&queue));
printf("Dequeue: %d\n", dequeue(&queue));
printf("Dequeue: %d\n", dequeue(&queue));
printf("Queue size: %d\n", getSize(&queue));
clearQueue(&queue);
printf("Queue size: %d\n", getSize(&queue));
free(queue.data);
return 0;
}
```
这段代码实现了循环队列的初始化、入队、出队、判空、判满、获取队头元素、获取队列长度和清空队列等基本操作。请根据自己的需要进行修改和完善。
相关推荐
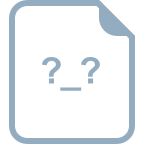
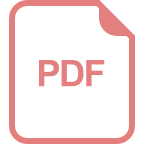















