给我用C-Sharp写一段在MAUI.Windows下的选择文件夹的函数
时间: 2023-09-16 10:05:33 浏览: 234
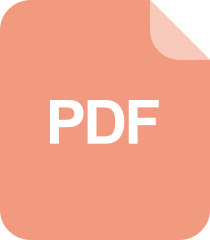
dotnet-maui-net-maui-8.0.pdf
### 回答1:
非常好。
您可以使用 Windows.Storage 命名空间中的 FolderPicker 类来选择文件夹。
具体地,您可以使用以下代码打开文件夹选择器并获取选定的文件夹:
```
FolderPicker folderPicker = new FolderPicker();
folderPicker.SuggestedStartLocation = PickerLocationId.Desktop;
folderPicker.FileTypeFilter.Add("*");
StorageFolder folder = await folderPicker.PickSingleFolderAsync();
```
您还可以使用 FileTypeFilter 属性来限制可以选择的文件类型。例如,要仅允许选择图像文件,您可以将其更改为:
```
folderPicker.FileTypeFilter.Add(".jpg");
folderPicker.FileTypeFilter.Add(".png");
folderPicker.FileTypeFilter.Add(".bmp");
```
希望这可以帮到您!
### 回答2:
在MAUI.Windows下,可以使用C#编写以下函数来选择文件夹:
```csharp
using Microsoft.Maui.Essentials;
using System.Threading.Tasks;
public static class FolderPicker
{
public static async Task<string> SelectFolder()
{
var result = await FilePicker.PickFolderAsync();
if (result != null)
{
return result.FullPath;
}
else
{
return string.Empty;
}
}
}
```
上述代码使用了`Microsoft.Maui.Essentials`命名空间中的`FilePicker`类,该类提供了选择文件夹的功能。在`SelectFolder()`方法中,使用`await FilePicker.PickFolderAsync()`方法来弹出文件夹选择器,并等待用户选择文件夹。选择完成后,将返回选择的文件夹路径。
如果用户取消选择或出现其他错误,`PickFolderAsync()`方法返回null。因此,在函数的最后,检查结果是否为空,如果是,则返回一个空字符串。
请注意,实际上,MAUI中的文件选择功能可能会根据不同的平台有所不同。因此,如果你希望在其他平台上使用MAUI跨平台应用程序,可能需要根据相应平台上的不同API进行调整和处理。
该函数只是一个简单的示例,你可以根据自己的需求和应用程序的特定要求进行相应的更改和扩展。
### 回答3:
当然可以给您提供一个使用C#编写的在MAUI.Windows下选择文件夹的函数。以下是一个基本的示例:
```csharp
using Microsoft.Maui.Controls;
using Microsoft.Maui.Essentials;
using System.Threading.Tasks;
public class FilePickerService
{
public async Task<string> PickFolder()
{
var result = await FilePicker.PickFolderAsync();
if (result != null)
{
return result.FullPath;
}
return string.Empty;
}
}
public class MainPage : ContentPage
{
public MainPage()
{
var pickFolderButton = new Button
{
Text = "选择文件夹"
};
pickFolderButton.Clicked += async (sender, e) =>
{
var filePickerService = new FilePickerService();
string folderPath = await filePickerService.PickFolder();
if (!string.IsNullOrEmpty(folderPath))
{
await DisplayAlert("选择的文件夹路径:", folderPath, "确定");
}
};
Content = new StackLayout
{
VerticalOptions = LayoutOptions.CenterAndExpand,
Children = { pickFolderButton }
};
}
}
```
在这个示例中,我们首先创建了一个 `FilePickerService` 类,用于封装文件夹选择的逻辑。在 `PickFolder` 方法中,我们调用了 `FilePicker.PickFolderAsync` 方法来打开文件夹选择器,并等待用户选择一个文件夹。如果用户选择了文件夹,我们将返回其完整路径;否则,返回一个空字符串。
然后,在 `MainPage` 类的构造函数中,我们创建了一个按钮 `pickFolderButton`,当用户点击该按钮时,会调用 `PickFolder` 方法,并根据返回的文件夹路径显示一个弹窗。
请注意,为了能够使用 `FilePicker`,您需要在项目中安装并引用 `Microsoft.Maui.Essentials` 包,并确保 `MAUI.Windows` 目标项目已正确配置。由于 `MAUI` 目前还处于开发阶段,具体配置可能会有所不同,请参考相关文档以确保代码能够正确运行。
阅读全文
相关推荐
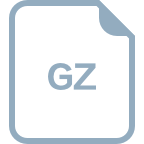
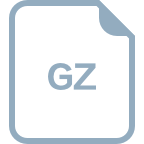















