我现在想大家一个网络,用stm32+9266,一共有三套这样的设备,其中一套作为ap,另外两套作为sta模式接入这个ap,请给出详细的代码
时间: 2024-09-23 19:03:57 浏览: 71
在STM32微控制器上配合ESP8266模块构建一个支持AP和STA模式的Wi-Fi网络系统,通常需要使用嵌入式WiFi库如Espressif IoT SDK。以下是粗略的示例代码结构,具体实现可能因所选的SDK、框架和库的不同而有所差异:
```c
#include "esp_wifi.h"
#include "esp_event_loop.h"
// AP配置
typedef struct {
char ssid[32]; // WiFi SSID
char password[32]; // WiFi密码
} ApConfig;
// STA配置
typedef struct {
char ap_ssid[32]; // 连接的AP SSID
char ap_password[32]; // AP的密码
char station_ssid[32]; // 自己设置的SSID用于STA模式
char station_password[32]; // Station模式下的密码
} StaConfig;
ApConfig ap_config = {"Your_AP_Name", "Your_AP_Pass"};
StaConfig sta_configs[2] = {{"Other_AP_Name", "Other_AP_Pass", "Station_SSID_1", "Station_Password_1"},
{"Other_AP_Name_2", "Other_AP_Pass_2", "Station_SSID_2", "Station_Password_2"}};
void wifi_ap(void)
{
esp_err_t err;
tcpip_adapter_init();
wifi_event_handler_register(WIFI_EVENT_AP_STA_CONNECTED, wifi_ap_connected);
wifi_event_handler_register(WIFI_EVENT_AP_STA_DISCONNECTED, wifi_ap_disconnected);
ESP_LOGI(TAG, "Setting up access point...");
err = esp_wifi_set_mode(WIFI_MODE_AP_STA);
if (err != ESP_OK) {
ESP_LOGE(TAG, "Failed to set mode AP_STA, error code: %d", err);
return;
}
err = esp_wifi_set_config(ESP_IF_WIFI_AP, &ap_config);
if (err != ESP_OK) {
ESP_LOGE(TAG, "Failed to configure AP, error code: %d", err);
return;
}
}
void wifi_ap_connected(void *ctx, system_event_t *event)
{
ESP_LOGI(TAG, "Connected to a station");
}
void wifi_ap_disconnected(void *ctx, system_event_t *event)
{
ESP_LOGI(TAG, "Disconnected from a station");
}
void wifi_sta(void)
{
for (int i = 0; i < 2; ++i) {
esp_netif_t *sta_if = esp_netif_create_default_standalone(&sta_configs[i].station_ssid, &sta_configs[i].station_password);
if (!sta_if) {
ESP_LOGE(TAG, "Failed to create sta interface");
continue;
}
err = esp_netif_start(sta_if);
if (err != ESP_OK) {
ESP_LOGE(TAG, "Failed to start sta interface, error code: %d", err);
esp_netif_destroy(sta_if);
continue;
}
esp_wifi_connect();
}
}
void app_main()
{
wifi_ap(); // 启动AP模式
wifi_sta(); // 遍历STA配置,尝试连接到其他AP
}
```
注意:这只是一个基本的示例,并未包含错误处理和状态检查。实际项目中你需要添加适当的错误处理代码以及网络状态检测(如定时检查是否成功连接)。同时,确保你的硬件连接正确并调整了必要的固件设置。
阅读全文
相关推荐
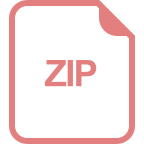
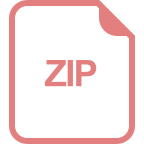
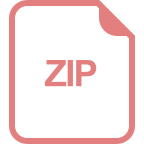
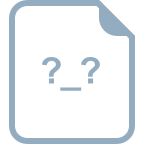
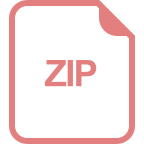
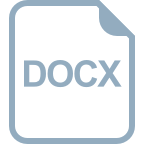
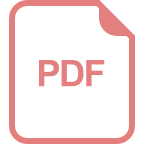
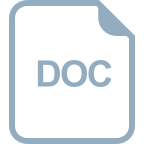
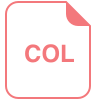

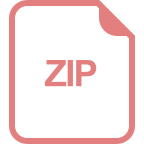
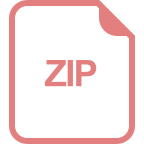
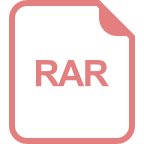
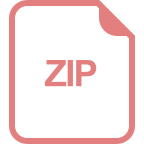
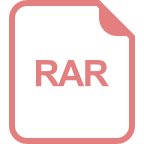
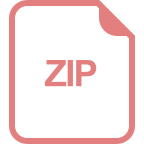