webrtc实现音视频通话的完整代码
时间: 2023-08-19 20:05:52 浏览: 63
抱歉,我并不是一个可以运行代码的程序,但是我可以指导您如何实现WebRTC的音视频通话。以下是一些步骤:
1. 首先,您需要使用WebRTC的JavaScript API。您可以在WebRTC官方网站上找到它们。
2. 实现信令服务器。WebRTC需要使用信令服务器来管理连接,包括建立和断开连接。您可以使用WebSocket或HTTP服务器来实现信令服务器。
3. 实现WebRTC连接。在创建连接之前,您需要使用信令服务器将连接信息传递给对方。当您的应用程序收到连接请求时,您需要创建一个RTCPeerConnection对象并将其添加到本地流中。
4. 实现音视频流。使用getUserMedia API来获取本地音视频流。将流添加到RTCPeerConnection对象中。
5. 实现呼叫控制。您需要为用户提供呼叫控制,例如开始和结束呼叫,以及静音和取消静音音频流。
6. 实现媒体协商。WebRTC使用媒体协商来确定哪些编解码器和参数使用。您需要编写代码来自动协商这些参数。
以上是WebRTC音视频通话的一般步骤,您可以根据您的具体需求进行修改和扩展。
相关问题
WebRTC实现音视频通话的代码
WebRTC 是一种用于实时通信的开放 Web 技术,支持音视频通话、文件传输等功能。以下是使用 WebRTC 实现音视频通话的代码示例:
1. 获取本地摄像头和麦克风
```javascript
navigator.mediaDevices.getUserMedia({
audio: true,
video: true
})
.then(stream => {
// stream 是获取到的本地音视频流
})
.catch(error => {
console.error(error);
});
```
2. 创建 RTCPeerConnection 对象
```javascript
const configuration = {
iceServers: [
{ urls: 'stun:stun.l.google.com:19302' },
{ urls: 'stun:stun1.l.google.com:19302' },
{ urls: 'stun:stun2.l.google.com:19302' }
]
};
const peerConnection = new RTCPeerConnection(configuration);
```
3. 添加本地流到 RTCPeerConnection 对象
```javascript
stream.getTracks().forEach(track => {
peerConnection.addTrack(track, stream);
});
```
4. 创建 Offer SDP
```javascript
peerConnection.createOffer().then(offer => {
return peerConnection.setLocalDescription(offer);
})
.then(() => {
// 获取 Offer SDP
const offerSdp = peerConnection.localDescription.sdp;
})
.catch(error => {
console.error(error);
});
```
5. 发送 Offer SDP 给远端
```javascript
// 使用 WebSocket 发送 Offer SDP 到远端
ws.send(JSON.stringify({
type: 'offer',
sdp: offerSdp
}));
```
6. 接收远端的 Answer SDP
```javascript
ws.onmessage = event => {
const message = JSON.parse(event.data);
if (message.type === 'answer') {
const answerSdp = message.sdp;
peerConnection.setRemoteDescription(new RTCSessionDescription({
type: 'answer',
sdp: answerSdp
}));
}
};
```
7. 创建 Answer SDP
```javascript
peerConnection.ontrack = event => {
// 获取远端音视频流
const remoteStream = event.streams[0];
};
peerConnection.createAnswer().then(answer => {
return peerConnection.setLocalDescription(answer);
})
.then(() => {
// 获取 Answer SDP
const answerSdp = peerConnection.localDescription.sdp;
})
.catch(error => {
console.error(error);
});
```
8. 发送 Answer SDP 给远端
```javascript
// 使用 WebSocket 发送 Answer SDP 到远端
ws.send(JSON.stringify({
type: 'answer',
sdp: answerSdp
}));
```
9. 接收 ICE 候选项
```javascript
ws.onmessage = event => {
const message = JSON.parse(event.data);
if (message.type === 'candidate') {
const candidate = new RTCIceCandidate({
sdpMLineIndex: message.label,
candidate: message.candidate
});
peerConnection.addIceCandidate(candidate);
}
};
```
10. 发送 ICE 候选项给远端
```javascript
peerConnection.onicecandidate = event => {
if (event.candidate) {
// 使用 WebSocket 发送 ICE 候选项到远端
ws.send(JSON.stringify({
type: 'candidate',
label: event.candidate.sdpMLineIndex,
id: event.candidate.sdpMid,
candidate: event.candidate.candidate
}));
}
};
```
以上是使用 WebRTC 实现音视频通话的基本代码示例,具体实现还需要根据业务需求进行调整。
vue+webrtc实现音视频通话
要使用Vue和WebRTC实现音视频通话,您可以使用WebRTC API。以下是一些步骤:
1. 首先,您需要创建一个新的Vue项目,您可以使用Vue CLI来创建。
2. 接下来,您需要使用WebRTC API来实现音视频通话。在Vue中,您可以使用Vue插件来轻松地使用WebRTC API。例如,您可以使用vue-webrtc插件。
3. 您需要在Vue组件中使用vue-webrtc插件。您可以在组件的模板中放置一个video标签,该标签将用于显示视频流。使用vue-webrtc插件,您可以轻松地与其他用户进行音视频通话。
4. 在Vue组件中,您可以使用WebRTC API来处理音视频流。您可以使用getUserMedia()函数获取本地视频流,并使用RTCPeerConnection对象将视频流发送到远程用户。使用RTCPeerConnection对象,您可以建立点对点连接,并在WebRTC流之间进行通信。
5. 最后,您需要使用Vue的事件来处理音视频通话中的各种情况,例如在连接断开时显示错误消息等。
这是一个简单的示例代码,演示如何使用Vue和WebRTC API实现音视频通话:
```
<template>
<div>
<video ref="localVideo" autoplay></video>
<video ref="remoteVideo" autoplay></video>
</div>
</template>
<script>
import VueWebRTC from 'vue-webrtc'
export default {
name: 'VideoChat',
components: {
VueWebRTC
},
data () {
return {
localStream: null,
remoteStream: null,
peerConnection: null
}
},
mounted () {
this.startLocalVideo()
},
methods: {
startLocalVideo () {
navigator.getUserMedia({ video: true, audio: true }, (stream) => {
this.localStream = stream
this.$refs.localVideo.srcObject = stream
this.setupPeerConnection()
}, (error) => {
console.error(error)
})
},
setupPeerConnection () {
this.peerConnection = new RTCPeerConnection()
this.peerConnection.onicecandidate = (event) => {
if (event.candidate) {
// send candidate to remote user
}
}
this.peerConnection.onaddstream = (event) => {
this.remoteStream = event.stream
this.$refs.remoteVideo.srcObject = event.stream
}
this.peerConnection.addStream(this.localStream)
// create offer and send to remote user
}
}
}
</script>
```
相关推荐
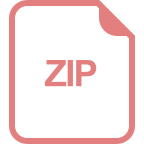
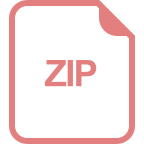












