public class StudentDemo { public static void main(String[] args) { //声明Student类数组(含3个元素) Student[] students = new Student[3]; Scanner in = new Scanner(System.in); //输入3个对象存入数组,其中一个为Student类的对象,一个StudentSJ类的对象,一个StudentZZ类的对象 students[0] = new Student(in.next(), in.next(), in.nextDouble(), in.nextDouble(), in.nextDouble()); students[1] = new StudentSJ(in.next(), in.next(), in.nextDouble(), in.nextDouble(), in.nextDouble(), in.next()); students[2] = new StudentZZ(in.next(), in.next(), in.nextDouble(), in.nextDouble(), in.nextDouble(), in.next()); //将方法testScore()发送给数组中的每一个元素,输出结果,并分析具体执行的是哪一个类中的方法 for (int i = 0; i < students.length; i++) { System.out.println(students[i].toString()); } System.out.println(); //比较StudentSJ类对象和StudentZZ类对象的总成绩,输出结果 if (students[1].compare(students[2]) > 0) { System.out.println( "'s total score is more than Wangwu"); } else if (students[1].compare(students[2]) == 0) { System.out.println("李四的总成绩和王五相同"); } else { System.out.println(students[1] + "'s total score is less than " + students[2]); } } }如何在输出时仅输出数组的某一项
时间: 2024-02-04 19:01:43 浏览: 59
如果你想在输出时仅输出数组的某一项,可以在输出时使用数组的下标来定位某一项,例如:
```java
System.out.println(students[1].getName() + "'s total score is less than " + students[2].getName());
```
这里使用了`students[1].getName()`和`students[2].getName()`来仅输出数组中第二项(`students[1]`)和第三项(`students[2]`)的姓名。你可以通过类似这样的方式来输出数组中的其他属性。
相关问题
public class TestPolymorphism { public static void main(String[] args) { // 声明Student类的数组 Student[] students = new Student[5]; // 生成五个对象存入数组 students[0] = new Student(80, "张三", 90, 99,100); students[1] = new Student(70, "李四", 90, 80, 80); students[2] = new Student(77, "王五", 70, 90, 100); students[3] = new StudentXW("小王", 85, null, 90, 99, 80); students[4] = new StudentBZ("小李", 95, null, 80, 90, 100); // 对数组中的每个元素都调用testscore()方法进行测试 for (int i = 0; i < students.length; i++) { students[i].testScore(); } } }怎么让他输出结果呢
这段代码的输出结果应该是每个学生的姓名以及总分和平均分。你可以在 `Student` 类的 `testScore()` 方法中添加输出语句来打印结果。
例如,在 `Student` 类的 `testScore()` 方法中,你可以添加以下代码来输出学生的姓名、总分和平均分:
```
System.out.println("姓名:" + name);
System.out.println("总分:" + getTotalScore());
System.out.println("平均分:" + getAverageScore());
```
注意,如果你想打印学生特有的属性(如 `StudentXW` 类的 `xwScore` 属性或 `StudentBZ` 类的 `bzScore` 属性),你需要将数组元素强制转换为相应的子类。例如,在循环中,你可以添加以下代码来打印 `StudentXW` 类型学生的 `xwScore` 属性:
```
if (students[i] instanceof StudentXW) {
StudentXW studentXW = (StudentXW) students[i];
System.out.println("小王的小学科目成绩:" + studentXW.getXwScore());
}
```
同样的,你也可以添加类似的代码来打印 `StudentBZ` 类型学生的 `bzScore` 属性。
请问下列语句1和语句2的information值分别是? class Student { int age; String name; public String toString(){ return "Student"; } } class Bachelor extends Student { String counselor;//辅导员 public String toString(){ return "Bachelor"; } } class Master extends Student { String supervisor;//硕士导师 public String toString(){ return "Master"; } } class Doctor extends Student { String supervisor;//博士导师 public String toString(){ return "Doctor"; } } public class Test { public static void main(String[] args) { Student tom=new Student(); Bachelor jack=new Bachelor(); Master rose=new Master(); Doctor jerry=new Doctor(); String informati""; tom=jack; informatitom.toString(); //语句1 tom=rose; informatitom.toString(); //语句2 } }
在语句1中,`informati`的值为"Bachelor",因为`tom`此时指向`jack`对象,而`jack`是`Bachelor`类的一个实例,所以调用`tom.toString()`实际上是调用`jack.toString()`,返回的是"Bachelor"。
在语句2中,`informati`的值为"Master",因为`tom`此时指向`rose`对象,而`rose`是`Master`类的一个实例,所以调用`tom.toString()`实际上是调用`rose.toString()`,返回的是"Master"。
阅读全文
相关推荐
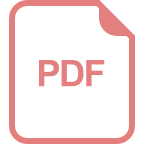
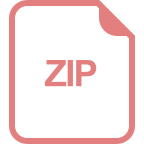
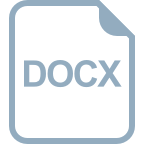









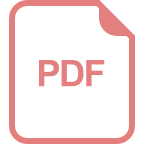