.net core 实现oauth2.0服务端,含JWT
时间: 2023-12-16 19:03:02 浏览: 46
要实现OAuth2.0服务端,需要遵循OAuth2.0协议,实现授权服务器和资源服务器之间的交互。以下是基本的步骤:
1.创建一个ASP.NET Core Web API项目。
2.安装IdentityServer4和Microsoft.AspNetCore.Authentication.JwtBearer NuGet包。
3.在Startup.cs中配置IdentityServer,并添加JwtBearer认证。
4.创建客户端和API资源,并将它们添加到IdentityServer中。
5.实现OAuth2.0授权终结点,例如授权码授权、密码授权、客户端凭证授权等。
6.实现API资源的保护,验证JWT令牌并确保访问令牌有效。
7.测试OAuth2.0授权和保护API资源。
下面是一个简单的示例,演示如何实现OAuth2.0服务端,包括JWT:
1. 在Startup.cs中配置IdentityServer和JwtBearer认证。示例代码如下:
```csharp
public void ConfigureServices(IServiceCollection services)
{
//添加IdentityServer服务
services.AddIdentityServer()
.AddInMemoryClients(Config.Clients)
.AddInMemoryApiResources(Config.Apis)
.AddDeveloperSigningCredential();
//添加JwtBearer认证
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.Authority = "http://localhost:5000"; //IdentityServer地址
options.RequireHttpsMetadata = false; //HTTPS设置为false,方便测试
options.Audience = "api1"; //API资源名称
});
services.AddControllers();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseRouting();
app.UseIdentityServer();
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
```
2. 创建客户端和API资源。示例代码如下:
```csharp
public static class Config
{
public static IEnumerable<Client> Clients => new List<Client>
{
new Client
{
ClientId = "client1",
ClientSecrets = { new Secret("secret1".Sha256()) },
AllowedGrantTypes = GrantTypes.ClientCredentials,
AllowedScopes = { "api1" }
}
};
public static IEnumerable<ApiResource> Apis => new List<ApiResource>
{
new ApiResource("api1", "My API")
};
}
```
3. 实现授权终结点。示例代码如下:
```csharp
[HttpPost]
[Route("/connect/token")]
public async Task<IActionResult> Token([FromBody] TokenRequest request)
{
if (request.GrantType == "password")
{
var user = _userService.ValidateCredentials(request.UserName, request.Password);
if (user != null)
{
var accessToken = await _tokenService.CreateAccessTokenAsync(user);
return Ok(new
{
access_token = accessToken,
token_type = "Bearer",
expires_in = (int)_tokenService.Options.Expiration.TotalSeconds
});
}
}
return BadRequest(new
{
error = "unsupported_grant_type"
});
}
```
4. 实现API资源的保护。示例代码如下:
```csharp
[Authorize]
[HttpGet]
[Route("test")]
public IActionResult Test()
{
return Ok(new
{
message = "Hello, World!"
});
}
```
以上是一个基本的OAuth2.0服务端实现,包括JWT。你可以根据自己的需求进行调整和扩展。
相关推荐
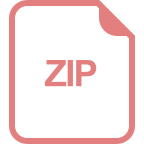
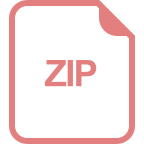













