springboot 下载邮件
时间: 2023-07-05 13:36:07 浏览: 110
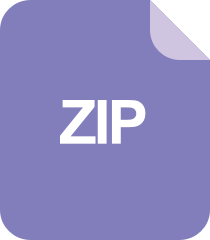
springboot邮件服务

要在Spring Boot中下载邮件,你可以使用JavaMail API来实现。以下是一个简单的示例代码,可以下载并保存邮件附件。
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Properties;
import javax.mail.BodyPart;
import javax.mail.Flags;
import javax.mail.Folder;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Multipart;
import javax.mail.Session;
import javax.mail.Store;
public class DownloadMailAttachments {
public static void main(String[] args) throws MessagingException, IOException {
String host = "mail.example.com";
String username = "username";
String password = "password";
String downloadDirectory = "/path/to/download/directory";
Properties properties = new Properties();
properties.setProperty("mail.store.protocol", "imaps");
Session session = Session.getInstance(properties, null);
Store store = session.getStore();
store.connect(host, username, password);
Folder inboxFolder = store.getFolder("INBOX");
inboxFolder.open(Folder.READ_WRITE);
Message[] messages = inboxFolder.getMessages();
System.out.println("Total Messages: " + messages.length);
for (int i = 0; i < messages.length; i++) {
Message message = messages[i];
String contentType = message.getContentType();
String subject = message.getSubject();
if (contentType.contains("multipart")) {
Multipart multiPart = (Multipart) message.getContent();
int numberOfParts = multiPart.getCount();
for (int partCount = 0; partCount < numberOfParts; partCount++) {
BodyPart bodyPart = multiPart.getBodyPart(partCount);
if (bodyPart.getDisposition() != null && bodyPart.getDisposition().equalsIgnoreCase("ATTACHMENT")) {
String fileName = bodyPart.getFileName();
InputStream inputStream = bodyPart.getInputStream();
Path filePath = Paths.get(downloadDirectory, fileName);
FileOutputStream outputStream = new FileOutputStream(filePath.toFile());
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
outputStream.close();
inputStream.close();
System.out.println("Attachment " + fileName + " downloaded from email subject: " + subject);
}
}
}
// Mark the message as deleted
message.setFlag(Flags.Flag.DELETED, true);
}
// expunge deleted messages
inboxFolder.close(true);
store.close();
}
}
```
你可以将上面的代码添加到你的Spring Boot应用程序中,然后替换`host`、`username`、`password`和`downloadDirectory`变量为相应的值,即可下载邮件。
阅读全文
相关推荐
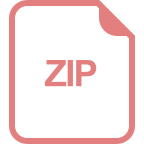
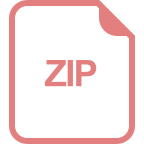
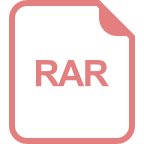
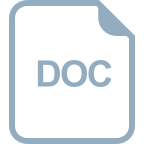

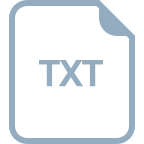
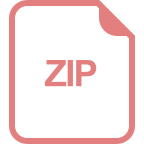
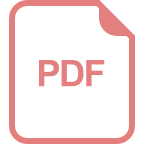
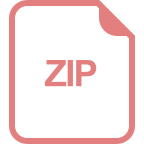
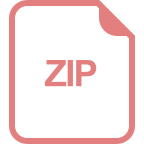
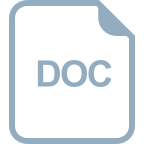
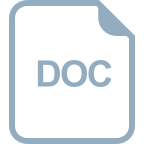
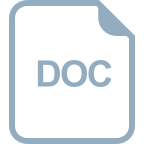

