用C++对图像进行掩模
时间: 2023-10-03 22:05:47 浏览: 51
在C语言中,我们可以使用位运算来对图像进行掩模操作。掩模操作可以通过将图像的每个像素与一个掩模图像的每个像素进行逐个比较,从而实现图像的局部区域处理。
以下是一个简单的例子,演示如何使用掩模对图像进行二值化处理:
```c
#include <stdio.h>
#include <stdlib.h>
#define BMP_HEADER_SIZE 54
typedef struct {
unsigned char blue;
unsigned char green;
unsigned char red;
} Pixel;
int main() {
FILE *input_file = fopen("input.bmp", "rb");
FILE *output_file = fopen("output.bmp", "wb");
if (input_file == NULL || output_file == NULL) {
printf("Error: Failed to open input/output file!\n");
exit(1);
}
// 读取位图文件头
unsigned char bmp_header[BMP_HEADER_SIZE];
fread(bmp_header, 1, BMP_HEADER_SIZE, input_file);
// 读取图像数据
int width = *(int *) (bmp_header + 18);
int height = *(int *) (bmp_header + 22);
int padding = (4 - ((width * sizeof(Pixel)) % 4)) % 4;
Pixel pixel, mask_pixel;
unsigned char mask[3][3] = {{ 0, 1, 0 },
{ 1, 1, 1 },
{ 0, 1, 0 }};
int sum;
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
sum = 0;
// 对于每个像素,计算掩模内像素的和
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
if (y + i < 0 || y + i >= height || x + j < 0 || x + j >= width) {
continue;
}
fseek(input_file, BMP_HEADER_SIZE + (y + i) * (width * sizeof(Pixel) + padding) + (x + j) * sizeof(Pixel), SEEK_SET);
fread(&pixel, sizeof(Pixel), 1, input_file);
mask_pixel.blue = mask[i + 1][j + 1] * pixel.blue;
mask_pixel.green = mask[i + 1][j + 1] * pixel.green;
mask_pixel.red = mask[i + 1][j + 1] * pixel.red;
sum += mask_pixel.blue + mask_pixel.green + mask_pixel.red;
}
}
// 根据掩模内像素的和决定当前像素的值
if (sum >= 127 * 3) {
pixel.blue = 255;
pixel.green = 255;
pixel.red = 255;
} else {
pixel.blue = 0;
pixel.green = 0;
pixel.red = 0;
}
// 写入输出文件
fseek(output_file, BMP_HEADER_SIZE + y * (width * sizeof(Pixel) + padding) + x * sizeof(Pixel), SEEK_SET);
fwrite(&pixel, sizeof(Pixel), 1, output_file);
}
}
fclose(input_file);
fclose(output_file);
return 0;
}
```
在这个例子中,我们使用了一个 3×3 的掩模,对图像进行了简单的二值化处理。当掩模内像素的和大于等于 127×3 时,当前像素的值被设为白色,否则被设为黑色。
你可以根据自己的需求修改掩模和处理的方式,实现更加复杂的图像处理操作。
相关推荐
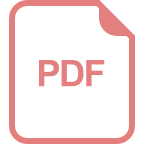
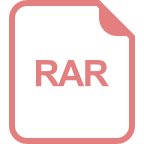
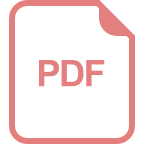
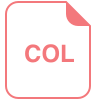













