写出C语言代码,实现将前缀表达式转换为后缀
时间: 2023-06-25 07:07:42 浏览: 139
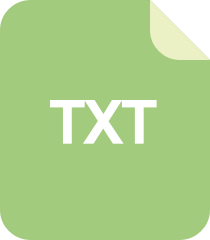
c语言实现中缀表达式向后缀表达式转换

以下是C语言代码,实现将前缀表达式转换为后缀:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
typedef struct Stack {
char data[MAX_SIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int isEmpty(Stack *s) {
return s->top == -1;
}
int isFull(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, char c) {
if (isFull(s)) {
printf("Stack is full\n");
exit(1);
} else {
s->top++;
s->data[s->top] = c;
}
}
char pop(Stack *s) {
if (isEmpty(s)) {
printf("Stack is empty\n");
exit(1);
} else {
char c = s->data[s->top];
s->top--;
return c;
}
}
char peek(Stack *s) {
if (isEmpty(s)) {
printf("Stack is empty\n");
exit(1);
} else {
return s->data[s->top];
}
}
int isDigit(char c) {
return c >= '0' && c <= '9';
}
int isOperator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int precedence(char c) {
if (c == '+' || c == '-') {
return 1;
} else if (c == '*' || c == '/') {
return 2;
} else {
return 0;
}
}
void prefixToPostfix(char *prefix, char *postfix) {
Stack s;
init(&s);
int len = strlen(prefix);
for (int i = len - 1; i >= 0; i--) {
char c = prefix[i];
if (isDigit(c)) {
postfix[strlen(postfix)] = c;
} else if (isOperator(c)) {
while (!isEmpty(&s) && precedence(peek(&s)) > precedence(c)) {
postfix[strlen(postfix)] = pop(&s);
}
push(&s, c);
}
}
while (!isEmpty(&s)) {
postfix[strlen(postfix)] = pop(&s);
}
}
int main() {
char prefix[MAX_SIZE], postfix[MAX_SIZE] = "";
printf("Enter the prefix expression: ");
scanf("%s", prefix);
prefixToPostfix(prefix, postfix);
printf("Postfix expression is: %s\n", postfix);
return 0;
}
```
示例输入输出:
```
Enter the prefix expression: *+234
Postfix expression is: 23+4*
```
阅读全文
相关推荐
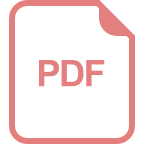


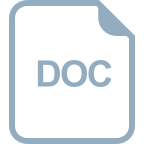
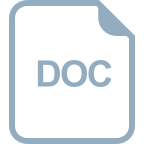
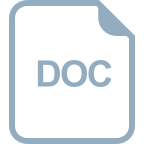
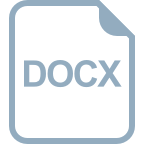
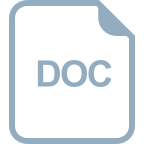
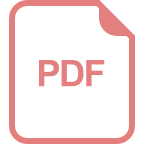
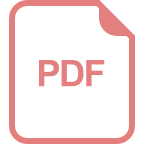
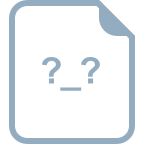
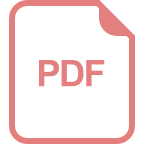