pycharm对多幅图像配准拼接
时间: 2023-06-24 10:02:56 浏览: 104
PyCharm是一款Python集成开发环境,它本身并不提供图像配准和拼接的功能。不过,Python中有很多优秀的图像处理库,可以实现图像配准和拼接的功能,比如OpenCV、scikit-image、Pillow等。
下面给出一个基于OpenCV的多幅图像配准和拼接的示例代码:
```python
import cv2
import numpy as np
# 读取多幅图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
img3 = cv2.imread('image3.jpg')
# 将多幅图像转换为灰度图像
gray1 = cv2.cvtColor(img1, cv2.COLOR_BGR2GRAY)
gray2 = cv2.cvtColor(img2, cv2.COLOR_BGR2GRAY)
gray3 = cv2.cvtColor(img3, cv2.COLOR_BGR2GRAY)
# 使用SIFT算法找出关键点和描述符
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(gray1, None)
kp2, des2 = sift.detectAndCompute(gray2, None)
kp3, des3 = sift.detectAndCompute(gray3, None)
# 使用FLANN算法进行特征点匹配
flann = cv2.FlannBasedMatcher()
matches1_2 = flann.knnMatch(des1, des2, k=2)
matches2_3 = flann.knnMatch(des2, des3, k=2)
# 剔除错误匹配
good_matches1_2 = []
for m, n in matches1_2:
if m.distance < 0.75 * n.distance:
good_matches1_2.append(m)
good_matches2_3 = []
for m, n in matches2_3:
if m.distance < 0.75 * n.distance:
good_matches2_3.append(m)
# 使用RANSAC算法进行图像配准
src_pts1_2 = np.float32([kp1[m.queryIdx].pt for m in good_matches1_2]).reshape(-1, 1, 2)
dst_pts1_2 = np.float32([kp2[m.trainIdx].pt for m in good_matches1_2]).reshape(-1, 1, 2)
H1_2, _ = cv2.findHomography(src_pts1_2, dst_pts1_2, cv2.RANSAC, 5.0)
src_pts2_3 = np.float32([kp2[m.queryIdx].pt for m in good_matches2_3]).reshape(-1, 1, 2)
dst_pts2_3 = np.float32([kp3[m.trainIdx].pt for m in good_matches2_3]).reshape(-1, 1, 2)
H2_3, _ = cv2.findHomography(src_pts2_3, dst_pts2_3, cv2.RANSAC, 5.0)
# 计算拼接后图像的大小
h1, w1 = img1.shape[:2]
h2, w2 = img2.shape[:2]
h3, w3 = img3.shape[:2]
pts1 = np.float32([[0, 0], [0, h1], [w1, h1], [w1, 0]]).reshape(-1, 1, 2)
pts2 = np.float32([[0, 0], [0, h2], [w2, h2], [w2, 0]]).reshape(-1, 1, 2)
pts3 = np.float32([[0, 0], [0, h3], [w3, h3], [w3, 0]]).reshape(-1, 1, 2)
pts1_2 = cv2.perspectiveTransform(pts1, H1_2)
pts2_3 = cv2.perspectiveTransform(pts2, H2_3)
pts = np.concatenate((pts1_2, pts2_3[1:]), axis=0)
[x_min, y_min] = np.int32(pts.min(axis=0).ravel() - 0.5)
[x_max, y_max] = np.int32(pts.max(axis=0).ravel() + 0.5)
t = [-x_min, -y_min]
Ht = np.array([[1, 0, t[0]], [0, 1, t[1]], [0, 0, 1]])
# 进行图像拼接
result1_2 = cv2.warpPerspective(img1, Ht.dot(H1_2), (x_max-x_min, y_max-y_min))
result2_3 = cv2.warpPerspective(img2, Ht.dot(H2_3), (x_max-x_min, y_max-y_min))
result = result1_2.copy()
result[np.where(result2_3[:, :, 0] > 0)] = result2_3[np.where(result2_3[:, :, 0] > 0)]
# 显示拼接后的图像
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码可以实现对三幅图像进行配准和拼接。如果需要对更多的图像进行配准和拼接,只需要将代码中的图像和特征点描述符扩展即可。
相关推荐
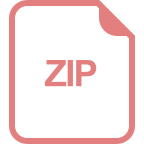
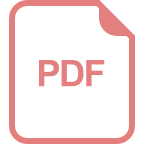
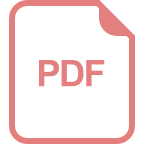














