写出时间片轮转调度算法的C语言代码,具体要求如下: 1.数据结构设计 PCB结构: name 进程名 round 进程轮转时间片 cputime 进程占用的CPU时间 needtime 进程到完成还要的时间 state 进程状态(假设状态为Ready、Run、Finish) next
时间: 2024-02-10 16:03:22 浏览: 34
这是一个简单的时间片轮转调度算法的C语言代码实现,代码中使用了链表来存储进程控制块(PCB):
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LENGTH 20
#define TIME_SLICE 2
// 进程状态定义
typedef enum {Ready, Run, Finish} State;
// 进程控制块(PCB)结构体
typedef struct pcb {
char name[MAX_NAME_LENGTH];
int round;
int cputime;
int needtime;
State state;
struct pcb *next;
} PCB;
// 初始化进程控制块
void init_pcb(PCB *pcb, char *name, int round, int needtime) {
strcpy(pcb->name, name);
pcb->round = round;
pcb->cputime = 0;
pcb->needtime = needtime;
pcb->state = Ready;
pcb->next = NULL;
}
// 插入进程控制块到链表尾部
void insert_pcb(PCB **head, PCB *pcb) {
if (*head == NULL) {
*head = pcb;
} else {
PCB *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = pcb;
}
}
// 从链表中删除进程控制块
void delete_pcb(PCB **head, PCB *pcb) {
if (*head == NULL) {
return;
}
if (*head == pcb) {
*head = pcb->next;
} else {
PCB *p = *head;
while (p->next != NULL && p->next != pcb) {
p = p->next;
}
if (p->next == pcb) {
p->next = pcb->next;
}
}
}
// 时间片轮转调度算法
void round_robin_scheduling(PCB **head) {
if (*head == NULL) {
return;
}
PCB *p = *head;
while (p != NULL) {
if (p->state == Run) {
p->cputime += TIME_SLICE;
p->needtime -= TIME_SLICE;
if (p->needtime <= 0) {
p->state = Finish;
printf("Process %s finished.\n", p->name);
}
else if (p->cputime >= p->round) {
p->state = Ready;
p->cputime = 0;
printf("Process %s time slice ended.\n", p->name);
}
break;
}
p = p->next;
}
if (p == NULL) {
p = *head;
} else {
p = p->next;
}
while (p != *head) {
if (p->state == Ready) {
p->state = Run;
printf("Process %s is running.\n", p->name);
break;
}
p = p->next;
}
if (p == *head && p->state != Run) {
printf("No process to run.\n");
}
}
// 打印进程状态
void print_state(PCB *pcb) {
switch (pcb->state) {
case Ready:
printf("Ready");
break;
case Run:
printf("Run");
break;
case Finish:
printf("Finish");
break;
}
}
// 打印所有进程状态
void print_all_state(PCB *head) {
PCB *p = head;
while (p != NULL) {
printf("Process %s, round %d, cputime %d, needtime %d, state ", p->name, p->round, p->cputime, p->needtime);
print_state(p);
printf("\n");
p = p->next;
}
}
int main() {
PCB *head = NULL;
PCB *p1 = (PCB *)malloc(sizeof(PCB));
init_pcb(p1, "Process1", 4, 10);
insert_pcb(&head, p1);
PCB *p2 = (PCB *)malloc(sizeof(PCB));
init_pcb(p2, "Process2", 2, 8);
insert_pcb(&head, p2);
PCB *p3 = (PCB *)malloc(sizeof(PCB));
init_pcb(p3, "Process3", 3, 6);
insert_pcb(&head, p3);
print_all_state(head);
printf("\n");
while(head != NULL) {
round_robin_scheduling(&head);
print_all_state(head);
printf("\n");
}
return 0;
}
```
这段代码实现了一个包含三个进程的时间片轮转调度算法。在运行时,程序会输出每个进程的状态,包括进程名、轮转时间片、已占用的CPU时间、剩余需要的CPU时间和状态(Ready、Run、Finish)。每次调度后,程序会输出当前正在运行的进程名和所有进程的状态。如果没有进程可以运行了,则输出“No process to run.”。
相关推荐
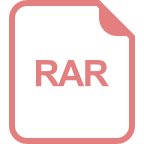














