public class GenericClass<T> { private List<T> list; public void printListType() { Type type = getClass().getGenericSuperclass(); ParameterizedType paramType = (ParameterizedType) type; Type[] argTypes = paramType.getActualTypeArguments(); Class<?> argClass = (Class<?>) argTypes[0]; Field[] fields = argClass.getDeclaredFields(); for (Field field : fields) { System.out.println(field.getType() + " " + field.getName()); } } } public class Main { public static void main(String[] args) { GenericClass<String> genericClass = new GenericClass<>(); genericClass.printListType(); } }
时间: 2024-03-10 11:48:13 浏览: 26
好的,可以使用 Hutool 优化这段代码。首先,需要导入 Hutool 的依赖包。然后,可以使用 Hutool 的 `TypeUtil` 类中的 `getTypeArgument()` 方法获取泛型类型。
优化后的代码如下所示:
```java
import cn.hutool.core.util.TypeUtil;
import java.lang.reflect.Field;
import java.lang.reflect.Type;
import java.lang.reflect.ParameterizedType;
import java.util.List;
public class GenericClass<T> {
private List<T> list;
public void printListType() {
Class<T> argClass = TypeUtil.getClass(this, 0);
Field[] fields = argClass.getDeclaredFields();
for (Field field : fields) {
System.out.println(field.getType() + " " + field.getName());
}
}
}
public class Main {
public static void main(String[] args) {
GenericClass<String> genericClass = new GenericClass<>();
genericClass.printListType();
}
}
```
在优化后的代码中,使用了 Hutool 的 `TypeUtil` 类的 `getClass()` 方法获取泛型类型,这样就不需要使用反射获取泛型类型了,代码更加简洁易读。
相关推荐
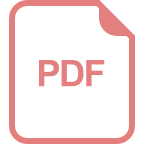
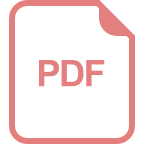
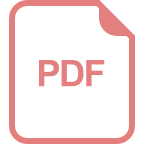















