编写一个计算机程序,用于计算给定公式的真值表 c++ (包含复制的逻辑运算)
时间: 2024-10-27 13:11:02 浏览: 17
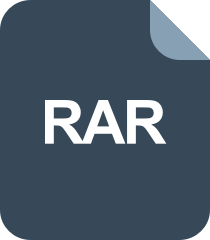
C++离散数学真值表的实现
为了编写一个C++程序,用于计算逻辑运算(如AND、OR、NOT)的真值表,你可以创建一个函数库,其中包含基本的逻辑操作,并创建一个主函数来遍历所有可能的输入组合并打印结果。以下是一个简单的示例:
```cpp
#include <iostream>
#include <bitset>
// 定义逻辑操作符
bool and_func(bool a, bool b) {
return a && b;
}
bool or_func(bool a, bool b) {
return a || b;
}
bool not_func(bool a) {
return !a;
}
// 真值表生成函数
void truth_table(const std::string& operator_name, int bits) {
for (int i = 0; i < (1 << bits); ++i) {
std::bitset<8> binary_i(i);
std::cout << "Input: (" << binary_i.to_string() << ") ";
if (operator_name == "and") {
std::cout << "Output: " << (and_func(binary_i.test(0), binary_i.test(1)) ? "True" : "False") << '\n';
} else if (operator_name == "or") {
std::cout << "Output: " << (or_func(binary_i.test(0), binary_i.test(1)) ? "True" : "False") << '\n';
} else if (operator_name == "not") {
std::cout << "Output: " << (not_func(binary_i.test(0)) ? "True" : "False") << '\n';
}
}
}
int main() {
// 示例:生成二进制位数为2的AND、OR和NOT运算的真值表
truth_table("and", 2);
truth_table("or", 2);
truth_table("not", 2);
return 0;
}
```
在这个例子中,我们首先定义了`and_func`、`or_func`和`not_func`三个函数分别对应逻辑与、或和非操作。然后,`truth_table`函数会生成指定操作和位数的真值表,打印出输入和对应的输出。
运行这个程序,它将显示每个逻辑操作在所有可能输入下的真值情况。
阅读全文
相关推荐
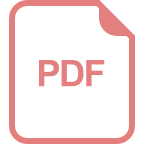
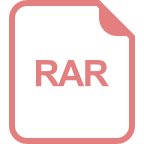


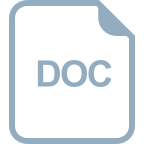
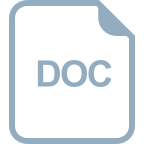
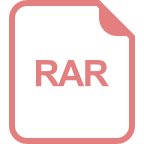
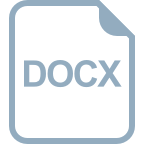
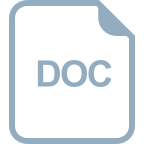
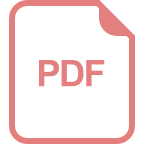
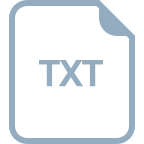
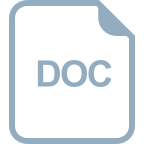
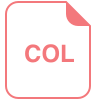
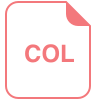
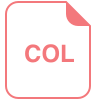