pta垂直直方图python
时间: 2023-10-05 10:14:24 浏览: 55
要制作 PTA(Power Trend Analysis)的垂直直方图,可以使用 Python 中的 matplotlib 库。以下是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
def plot_vertical_histogram(data):
# 计算直方图的数据
hist, bins = np.histogram(data, bins=10)
# 绘制垂直直方图
plt.barh(range(len(hist)), hist, align='center')
plt.yticks(range(len(hist)), bins[:-1])
# 添加标签和标题
plt.xlabel('Frequency')
plt.ylabel('Bins')
plt.title('Vertical Histogram')
# 显示图形
plt.show()
# 示例数据
data = [1, 2, 3, 4, 5, 5, 5, 6, 6, 6, 6, 7, 7, 8, 9]
# 绘制垂直直方图
plot_vertical_histogram(data)
```
这段代码将数据 `[1, 2, 3, 4, 5, 5, 5, 6, 6, 6, 6, 7, 7, 8, 9]` 绘制成一个垂直直方图。你可以根据自己的数据进行修改和调整。
相关问题
pta小猴吃桃python
PTA(浙江大学程序设计能力考试)是一种用于测试编程能力的在线评测系统。PTA小猴吃桃是PTA上的一道题目,下面是关于该题目的Python解法:
题目描述:
小猴子第一天摘下若干个桃子,当即吃了一半,还不过瘾,又多吃了一个。第二天早上又将剩下的桃子吃掉一半,又多吃了一个。以后每天早上都吃了前一天剩下的一半零一个。到第n天早上想再吃时,见只剩下一个桃子了。请问第一天共摘了多少个桃子?
输入格式:
输入包含一个整数n,表示天数。
输出格式:
输出包含一个整数,表示第一天共摘了多少个桃子。
示例输入:
3
示例输出:
10
解题思路:
根据题目描述,可以得出递推关系式:peach = (peach + 1) * 2
其中,peach表示第一天摘的桃子数量。根据递推关系式,可以使用循环来计算第一天摘的桃子数量。
Python代码如下:
```python
n = int(input())
peach = 1
for i in range(n-1):
peach = (peach + 1) * 2
print(peach)
```
PTA计算四面体体积python
PTA(Programming Teaching Assistant)是一个在线编程练习平台,用于帮助学生提高编程能力。计算四面体体积是一个常见的数学问题,可以使用Python编程语言来解决。
计算四面体体积的公式是:V = (1/6) * A * h,其中A是底面积,h是高。
下面是一个使用Python编程计算四面体体积的示例代码:
```python
import math
def calculate_tetrahedron_volume(a, h):
volume = (1/6) * a * h
return volume
# 输入底面积和高
base_area = float(input("请输入四面体的底面积:"))
height = float(input("请输入四面体的高:"))
# 调用函数计算体积
volume = calculate_tetrahedron_volume(base_area, height)
# 输出结果
print("四面体的体积为:", volume)
```
你可以在PTA平台上创建一个题目,要求学生编写类似的代码来计算四面体的体积,并进行自动评测。
相关推荐
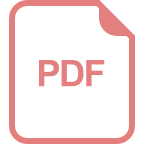
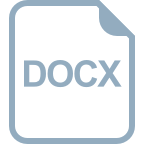












