企业微信机器人图文消息,C#代码
时间: 2023-11-13 18:44:11 浏览: 143
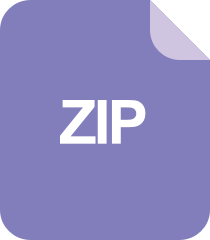
一个能够将消息推送至微信的机器人,基于Python和企业微信实现.zip
以下是使用 C# 代码向企业微信机器人发送图文消息的示例:
```csharp
using System;
using System.Collections.Generic;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
namespace WeChatRobotDemo
{
class Program
{
static async Task Main(string[] args)
{
// 企业微信机器人的 Webhook 地址
string webhookUrl = "https://qyapi.weixin.qq.com/cgi-bin/webhook/send?key=机器人的 key";
// 构建图文消息
List<Article> articles = new List<Article>()
{
new Article()
{
title = "标题1",
description = "描述1",
url = "http://www.example.com/article1.html",
picurl = "http://www.example.com/article1.png"
},
new Article()
{
title = "标题2",
description = "描述2",
url = "http://www.example.com/article2.html",
picurl = "http://www.example.com/article2.png"
}
};
NewsMessage message = new NewsMessage()
{
articles = articles
};
// 将消息转换为 JSON 字符串
string json = JsonConvert.SerializeObject(message);
// 发送 HTTP POST 请求
using (HttpClient httpClient = new HttpClient())
{
StringContent content = new StringContent(json, Encoding.UTF8, "application/json");
HttpResponseMessage response = await httpClient.PostAsync(webhookUrl, content);
if (response.IsSuccessStatusCode)
{
Console.WriteLine("消息发送成功");
}
else
{
Console.WriteLine($"消息发送失败,错误码:{response.StatusCode}");
}
}
}
}
class NewsMessage
{
public string msgtype { get; set; } = "news";
public List<Article> articles { get; set; }
}
class Article
{
public string title { get; set; }
public string description { get; set; }
public string url { get; set; }
public string picurl { get; set; }
}
}
```
注意事项:
- 机器人的 Webhook 地址需要替换为自己的地址,具体获取方法可以参考企业微信官方文档。
- 图片链接需要是公网可访问的 URL 地址,否则无法正常显示。
阅读全文
相关推荐
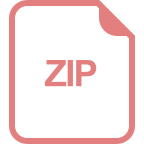
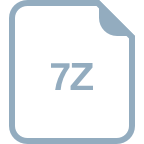
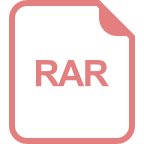
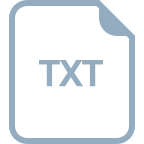
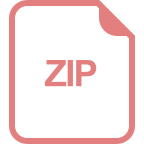
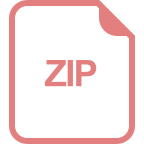
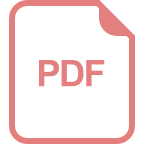
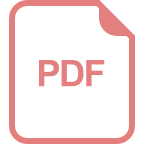
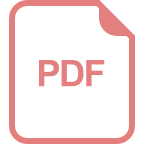
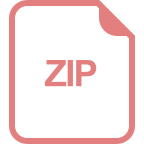
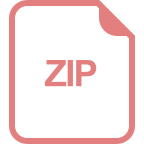
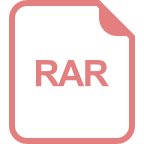
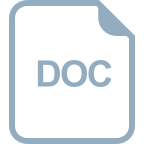
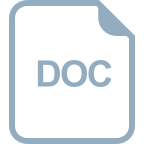
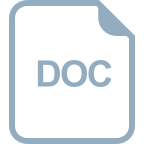
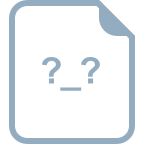