用c++输⼊⼀个字符串,将字符串中⼤写字⺟全部转为⼩写字⺟,⼩写字⺟转成⼤写字⺟,其他字符保持不 变。注:字符串的⻓度⼩于等于30,其中字符串中包含空格
时间: 2024-10-12 09:08:27 浏览: 14
在C++中,你可以通过使用标准库中的`std::transform`函数以及`std::isalpha`、`std::toupper`和`std::tolower`函数来实现这个功能。这里是一个简单的示例:
```cpp
#include <iostream>
#include <string>
#include <algorithm>
#include <cctype>
std::string swap_case(std::string input) {
std::transform(input.begin(), input.end(), input.begin(), ::tolower); // 转换小写字母
for (char& c : input) {
if (std::isupper(c)) { // 检查是否为大写字母
c = ::toupper(c); // 如果是,则转换为大写
}
}
return input;
}
int main() {
std::string str;
std::cout << "请输入一个字符串(长度小于等于30,可能包含空格): ";
getline(std::cin, str);
std::string result = swap_case(str);
std::cout << "转换后的字符串: " << result << "\n";
相关问题
用c++输⼊⼀个字符串,将字符串中⼤写字⺟全部转为⼩写字⺟,⼩写字⺟转成⼤写字⺟,其他字符保持不 变。注:字符串的⻓度⼩于等于30,其中字符串中包含空格
在C++中,你可以使用标准库提供的`<algorithm>`和`<cctype>`头文件中的函数来实现这个功能。下面是一个简单的示例程序:
```cpp
#include <iostream>
#include <string>
#include <algorithm>
#include <cctype>
std::string toggle_case(std::string str) {
std::transform(str.begin(), str.end(), str.begin(), ::tolower); // 转换为小写
for (size_t i = 0; i < str.size(); i++) { // 然后遍历字符串,遇到非字母字符再转换回大写
if (isalpha(str[i])) {
if (islower(str[i])) {
str[i] = toupper(str[i]); // 如果是小写字母,则转为大写
} else if (isupper(str[i])) {
str[i] = tolower(str[i]); // 否则,如果是大写字母,转为小写
}
}
}
return str;
}
int main() {
std::string input;
std::cout << "请输入一个字符串(长度小于等于30,包含空格):";
getline(std::cin, input);
std::string result = toggle_case(input);
std::cout << "处理后的字符串:\n" << result << std::endl;
return 0;
}
```
在这个程序里,`toggle_case`函数接收一个字符串作为输入,首先通过`std::transform`函数将其转换为全小写。然后它遍历字符串,当遇到字母时判断其当前状态并相应地切换大小写。
注意,这里假设输入字符串不会超过30个字符,并且包含空格。实际应用中,为了更健壮地处理这种情况,可以添加适当的错误检查和边界条件检查。
用c++定义一个字符串,分别统计该字符串中大写字母、小写字母和数字字符的个数,并输出统计结果(利用函数实现) 如输入字符串: ABab123cdAB,则大写字 母的个数为4,小写字母 的个数为4,数字字符的 个数为3.
以下是用C++函数实现的代码:
```c++
#include <iostream>
#include <string>
using namespace std;
void count(string str, int &upper, int &lower, int &digit)
{
for(char c : str)
{
if(c >= 'A' && c <= 'Z')
upper++;
else if(c >= 'a' && c <= 'z')
lower++;
else if(c >= '0' && c <= '9')
digit++;
}
}
int main()
{
string str = "ABab123cdAB";
int upper = 0, lower = 0, digit = 0;
count(str, upper, lower, digit);
cout << "大写字母的个数为" << upper << endl;
cout << "小写字母的个数为" << lower << endl;
cout << "数字字符的个数为" << digit << endl;
return 0;
}
```
输出结果为:
```
大写字母的个数为4
小写字母的个数为4
数字字符的个数为3
```
阅读全文
相关推荐
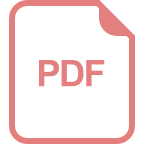
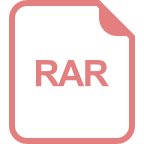
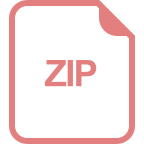
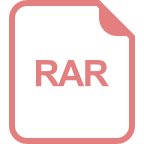
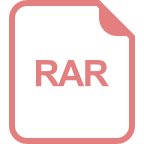
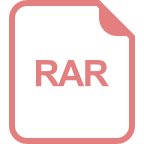
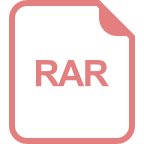
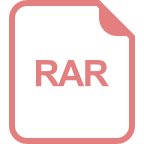
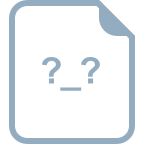
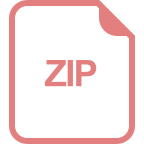
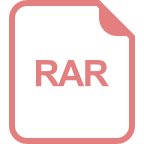
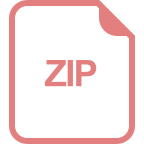
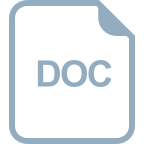
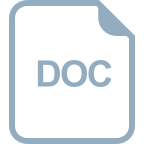
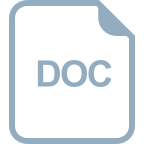

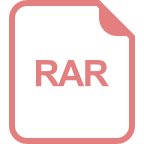