@Aspect Spring
时间: 2023-11-03 08:59:40 浏览: 37
@Aspect is an annotation in Spring that is used to define an aspect, which is a modularization of a concern that cuts across multiple classes. Aspects are used to encapsulate cross-cutting functionalities, such as logging, security, transaction management, and error handling, and apply them to multiple classes in a consistent and reusable manner.
To use @Aspect, you need to define an aspect class that contains advice and pointcut expressions. The advice defines the action to be taken when a particular pointcut expression is matched, while the pointcut expression defines the set of join points where the advice should be applied.
Here is an example of an aspect class that logs the execution of a method:
```
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.MyService.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Method " + joinPoint.getSignature().getName() + " is about to be executed...");
}
@AfterReturning("execution(* com.example.MyService.*(..))")
public void logAfterReturning(JoinPoint joinPoint) {
System.out.println("Method " + joinPoint.getSignature().getName() + " has been executed successfully.");
}
@AfterThrowing("execution(* com.example.MyService.*(..))")
public void logAfterThrowing(JoinPoint joinPoint) {
System.out.println("Method " + joinPoint.getSignature().getName() + " has thrown an exception.");
}
}
```
In this example, the aspect class logs the execution of any method in the com.example.MyService class. The @Before advice is executed before the method is executed, the @AfterReturning advice is executed after the method has returned successfully, and the @AfterThrowing advice is executed after the method has thrown an exception.
To enable the aspect in your Spring application, you need to add the @EnableAspectJAutoProxy annotation to your configuration class. This annotation enables Spring's aspect-oriented programming (AOP) support and allows the framework to create proxies for the classes that match the pointcut expressions defined in the aspect.
```
@Configuration
@EnableAspectJAutoProxy
public class AppConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
@Bean
public LoggingAspect loggingAspect() {
return new LoggingAspect();
}
}
```
In this example, the AppConfig class defines a bean for the MyService interface and its implementation, as well as a bean for the LoggingAspect class. The @EnableAspectJAutoProxy annotation enables AOP support in the application context and allows Spring to intercept calls to the MyService bean and apply the logging aspect to it.
相关推荐
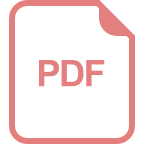
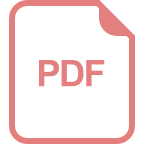














