pycharm中切换至 db_article 数据库 操作t_product集合 1.在控制台展示t_product集合 所有文档的商品名称,单价,类型信息。 2.插入一条商品信息(商品信息包含商品名称,单价,类型) 3.依据商品名称更改商品单价 4.根据商品名称删除商品信息
时间: 2024-09-30 09:15:04 浏览: 35
在PyCharm中操作MongoDB数据库(假设db_article是一个已经连接好的数据库,t_product是其中的一个集合),你可以按照以下步骤来完成你的需求:
1. **展示所有文档的商品信息:**
```python
from pymongo import MongoClient
# 连接到db_article数据库
client = MongoClient('mongodb://localhost:27017/')
db = client.db_article
collection = db.t_product
# 查询并显示所有文档的商品名称、单价和类型
for product in collection.find():
print(f"商品名称: {product['name']}, 单价: {product['price']}, 类型: {product['type']}")
```
2. **插入商品信息:**
```python
new_product = {
"name": "新商品名",
"price": 99.99,
"type": "电子产品"
}
collection.insert_one(new_product)
print("商品已成功插入.")
```
3. **更改商品单价:**
```python
update_result = collection.update_one({"name": "新商品名"}, {"$set": {"price": 109.99}})
if update_result.matched_count > 0:
print(f"商品名称为'{new_product['name']}' 的单价已更新为109.99")
else:
print("未找到匹配的商品进行单价更新.")
```
4. **删除商品信息:**
```python
delete_result = collection.delete_one({"name": "新商品名"})
if delete_result.deleted_count > 0:
print(f"商品名称为'{new_product['name']}' 的记录已被删除.")
else:
print("未找到该商品进行删除.")
```
记得在实际操作前确认数据库连接设置和权限,并根据实际情况修改数据库地址、集合名以及商品信息。
阅读全文
相关推荐















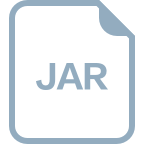

