如何使用 Lua 语言编写代码来判断一个字符串是否只包含数字、字母,或者是数字和字母的组合?
时间: 2024-12-12 17:29:46 浏览: 17
在 Lua 中,你可以使用标准库函数 `string` 来检查字符串的内容。下面是一些步骤:
1. **检查是否全由数字组成**:
使用 `string.match()` 函数和正则表达式 `\d+`,该模式匹配一串连续的数字。如果返回值非空,则说明字符串仅由数字组成。
```lua
function is_digits_only(str)
return str and string.match(str, "^%d+$") ~= nil
end
```
2. **检查是否全由字母组成**:
同样,使用正则表达式 `\w+`,`\w` 匹配任意字母、数字或下划线。如果匹配成功,则字符串仅由字母组成。
```lua
function is_letters_only(str)
return str and string.match(str, "^[%a_]+$") ~= nil
end
```
3. **检查是否是数字和字母的组合**:
这需要两个条件同时满足,可以结合上面两个函数。
```lua
function is_alphanumeric(str)
return is_digits_only(str) or is_letters_only(str)
end
```
相关问题
c语言编写程序:读入空心等腰梯形的高度(也是首行字母数)和空心等腰梯形起始字母,然后输出空心字符等腰梯形,只读入起始字母和等腰梯形的高度,空心等腰梯形是指:首尾两行是由字母组成,中间行数均为两端为字母,中间为空格的字符串组成,每行的字符串中心对齐,两行字符串长度差2,且字母从给定的起始字母逐一递增
以下是 C 语言编写程序读入空心等腰梯形的高度和起始字母,然后输出空心字符等腰梯形的代码:
```
#include <stdio.h>
int main() {
int height;
char start;
printf("请输入等腰梯形的高度和起始字母,中间用空格分隔:\n");
scanf("%d %c", &height, &start);
// 输出首行
for (int i = 0; i < height; i++) {
printf("%c", start+i);
}
printf("\n");
// 输出中间行
for (int i = 2; i < height; i++) {
for (int j = 0; j < height-i; j++) {
printf(" ");
}
printf("%c", start+i-1);
for (int j = 0; j < 2*i-3; j++) {
printf(" ");
}
printf("%c\n", start+i);
}
// 输出末行
for (int i = 0; i < height; i++) {
printf("%c", start+height-1-i);
}
printf("\n");
return 0;
}
```
第二个问题:lua closure factory 完整代码。
以下是 Lua 编写的 closure factory 完整代码:
```
function make_counter()
-- 定义局部变量 count
local count = 0
-- 定义函数 inc_count,实现 count 的自增
local function inc_count()
count = count + 1
return count
end
-- 返回函数 inc_count
return inc_count
end
-- 实例化一个 counter
counter = make_counter()
-- 使用 counter 自增计数器并输出
for i = 1, 10 do
print(counter())
end
```
这段代码利用了 Lua 中的闭包特性,实现了一个计数器,每次调用 counter 函数就会将 count 自增并返回新的计数值。make_counter 函数返回的是一个函数,它维护了一个局部变量 count,这个变量只在该函数内有效,其他的函数不能访问。因此,每个实例化的 counter 都拥有独立的计数器。
编写函数fun2实现字符串加密,加密规则为:如果是字母,将其进行大小写转换;如果是数字,将其加8后,再和4整数;如果是其他字符,不变。从键盘上输入字符串,调用该函数实现加密功能。
好的,以下是函数fun2的代码实现:
```lua
function fun2(str)
local result = ""
for i = 1, #str do
local c = string.sub(str, i, i)
if c >= "a" and c <= "z" then
result = result .. string.upper(c)
elseif c >= "A" and c <= "Z" then
result = result .. string.lower(c)
elseif c >= "0" and c <= "9" then
local num = tonumber(c)
num = num + 8
num = num % 4
result = result .. tostring(num)
else
result = result .. c
end
end
return result
end
```
你可以通过以下代码调用该函数:
```lua
local str = io.read()
local encryptedStr = fun2(str)
print(encryptedStr)
```
输入一个字符串后,程序会输出加密后的字符串。
阅读全文
相关推荐
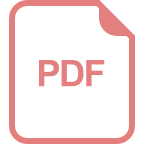
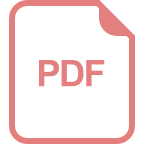
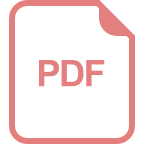
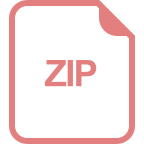
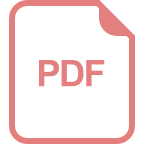
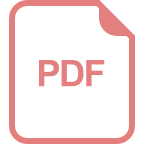
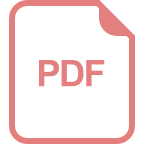
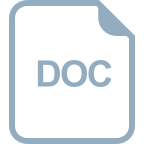
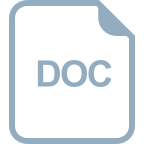
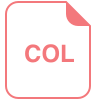
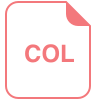





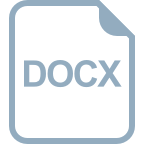