请结合数据结构实现简单的学生信息管理,包括学生姓名,学号后四位,年龄,性别,三门课成绩,录入学生信息时能够不按照任何顺序进行录入,能够支持后续任意时刻的信息录入。成绩的录入要求能够按照学号顺序进行批量录入,并在录入时打印学生信息。要求按照顺序结构实现。c语言
时间: 2024-11-06 15:15:12 浏览: 30
在C语言中,我们可以使用链表数据结构来实现这种简单的学生信息管理。链表的动态特性使得它可以方便地插入和删除元素,不需要预先确定元素的数量。这里是一个简单的示例,使用了结构体(struct)、节点(Node)和链表(LinkedList)的概念:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生信息结构体
typedef struct {
char name[50];
char id[10];
int age;
char gender;
float scores[3]; // 三门课的成绩
} Student;
// 链表节点
typedef struct Node {
Student info;
struct Node* next;
} Node;
// 创建链表头结点
Node* createList() {
return (Node*)malloc(sizeof(Node));
}
// 插入学生信息到链表末尾
void insert(Student* student, Node** head) {
Node* newNode = createList();
newNode->info = *student;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 批量录入成绩并打印学生信息
void batchInsert(int numStudents, Node** head) {
for (int i = 0; i < numStudents; i++) {
printf("请输入学生 %d 的信息(按顺序,例如:张三 1234 18 M 85.5 90.0 75.0):", i + 1);
scanf("%s %s %d %c %f %f %f", &(*head)->info.name, &(*head)->info.id, &(*head)->info.age, &(*head)->info.gender, &(*head)->info.scores[0], &(*head)->info.scores[1], &(*head)->info.scores[2]);
printf("\n");
}
}
// 打印链表中的所有学生信息
void printList(Node* head) {
while (head != NULL) {
printf("姓名:%s, 学号后四位:%s, 年龄:%d, 性别:%c, 成绩:%0.1f-%0.1f-%0.1f\n",
head->info.name, head->info.id, head->info.age, head->info.gender,
head->info.scores[0], head->info.scores[1], head->info.scores[2]);
head = head->next;
}
}
int main() {
Node* head = createList();
int numStudents;
printf("请输入学生总数:");
scanf("%d", &numStudents);
batchInsert(numStudents, &head);
printList(head);
free(head); // 释放内存
return 0;
}
```
这个程序会提示用户输入学生数量,然后依次录入每个学生的详细信息,包括姓名、学号后四位、年龄、性别和三门课成绩。最后,它会打印出链表中的所有学生信息。
阅读全文
相关推荐
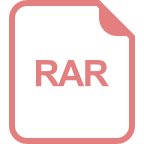
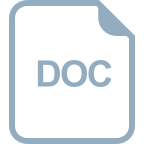
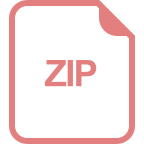





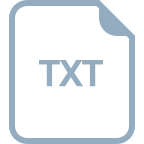
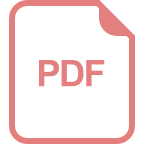
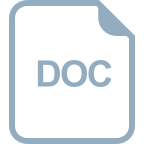
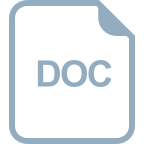
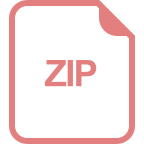
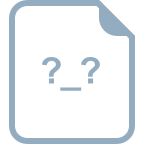
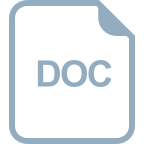