Qt只读取txt文件中的中文字符
时间: 2023-09-09 16:07:20 浏览: 43
Qt读取文本文件中的中文字符与其他字符一样,没有特殊的限制或要求。您可以使用以下代码读取文本文件,并确保正确设置文件编码:
```cpp
QString fileName = "your_file.txt";
QFile file(fileName);
if (!file.open(QIODevice::ReadOnly | QIODevice::Text)) {
// 文件打开失败
return;
}
QTextStream in(&file);
in.setCodec("UTF-8"); // 设置文件编码
while (!in.atEnd()) {
QString line = in.readLine();
// 处理每一行文本,包括中文字符
}
file.close();
```
在上述代码中,`in.setCodec("UTF-8")` 语句将文件编码设置为 UTF-8,以确保正确读取中文字符。如果您的文本文件使用其他编码格式,您需要相应地更改该语句。
相关问题
Qt只读取txt文件中的中文字符不读取数字
可以使用Qt的QString类和正则表达式来实现这个功能。首先,使用QFile类打开txt文件,然后读取文件内容,将其存储到一个QString对象中。接下来,使用正则表达式来匹配中文字符,并将其存储到另一个QString对象中。代码示例如下:
```cpp
#include <QFile>
#include <QTextStream>
#include <QString>
#include <QRegExp>
int main()
{
QFile file("test.txt");
if (!file.open(QIODevice::ReadOnly | QIODevice::Text))
return 1;
QTextStream in(&file);
QString content = in.readAll();
QRegExp rx("[\\u4e00-\\u9fa5]+"); // 正则表达式匹配中文字符
QStringList list;
int pos = 0;
while ((pos = rx.indexIn(content, pos)) != -1) {
list << rx.cap(0);
pos += rx.matchedLength();
}
qDebug() << list; // 输出中文字符列表
return 0;
}
```
这段代码会输出txt文件中所有的中文字符。如果需要排除数字,可以修改正则表达式,如下所示:
```cpp
QRegExp rx("[^0-9\\u4e00-\\u9fa5]+"); // 匹配除数字和中文字符以外的字符
```
这样就可以只获取中文字符了。
qt 读取本地文本文件,中文字符显示乱码,怎么解决
如果在Qt中读取本地文本文件出现了中文字符显示乱码的问题,可能是因为文件编码方式与Qt程序默认编码方式不一致所致。下面介绍两种解决方法:
1. 使用 QTextCodec 进行转换
可以使用 QTextCodec 类中的 fromUnicode() 和 toUnicode() 函数来进行编码转换。首先读取本地文件的原始数据,然后使用 fromUnicode() 函数将其转换为 QString 类型,最后再使用 toUtf8() 函数将其转换为 UTF-8 编码的 QByteArray 类型,示例如下:
```cpp
QFile file("your_file_path");
if (file.open(QIODevice::ReadOnly | QIODevice::Text))
{
QByteArray data = file.readAll();
QTextCodec *codec = QTextCodec::codecForName("GBK"); // 或者 "UTF-8",根据实际情况选择编码方式
QString str = codec->toUnicode(data);
QByteArray utf8_data = str.toUtf8();
// 处理 utf8_data 中的数据
file.close();
}
```
2. 修改 QTextStream 的编码方式
另一种解决方法是修改 QTextStream 的编码方式为本地文件的编码方式。示例如下:
```cpp
QFile file("your_file_path");
if (file.open(QIODevice::ReadOnly | QIODevice::Text))
{
QTextStream in(&file);
QTextCodec *codec = QTextCodec::codecForName("GBK"); // 或者 "UTF-8",根据实际情况选择编码方式
in.setCodec(codec);
QString str = in.readAll();
// 处理 str 中的数据
file.close();
}
```
以上两种方法都可以解决中文字符乱码的问题,具体使用哪种方法可以根据实际情况选择。
相关推荐
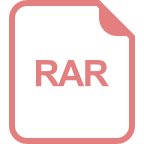












