输入一个年份和一个月份,判断并输出该月有多少天。(使用Switch语句实现)要求用户可以直接输入
时间: 2024-09-27 09:15:31 浏览: 29
要使用`Switch`语句来判断给定的年份和月份,你需要考虑闰年和平年的规则以及不同月份的天数。以下是一个简单的Java程序,它实现了这个功能:
```java
import java.util.Scanner;
public class MonthDaysCalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入年份:");
int year = scanner.nextInt();
System.out.println("请输入月份(1-12):");
int month = scanner.nextInt();
// 判断是否为闰年(根据四年一闰、百年不闰、四百年又闰的原则)
boolean isLeapYear = (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
// 切换语句判断每个月份的天数
switch (month) {
case 1:
System.out.println(month + "月有" + (isLeapYear ? 29 : 28) + "天");
break;
case 2:
System.out.println(month + "月有" + (isLeapYear ? 29 : 28) + "天");
break;
case 3:
System.out.println(month + "月有" + 31 + "天");
break;
case 4:
System.out.println(month + "月有" + 30 + "天");
break;
case 5:
System.out.println(month + "月有" + 31 + "天");
break;
case 6:
System.out.println(month + "月有" + 30 + "天");
break;
case 7:
System.out.println(month + "月有" + 31 + "天");
break;
case 8:
System.out.println(month + "月有" + 31 + "天");
break;
case 9:
System.out.println(month + "月有" + 30 + "天");
break;
case 10:
System.out.println(month + "月有" + 31 + "天");
break;
case 11:
System.out.println(month + "月有" + 30 + "天");
break;
case 12:
System.out.println(month + "月有" + (isLeapYear ? 31 : 30) + "天");
break;
default:
System.out.println("错误!月份应介于1到12之间.");
}
scanner.close();
}
}
```
在这个程序中,首先获取用户的输入,然后用`switch`语句检查月份并计算相应天数。如果月份大于12或小于1,会显示错误信息。
相关推荐
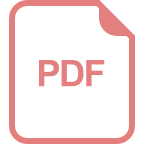
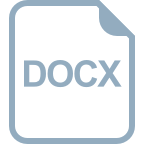














