在VS2017中用C#写一个类,这个类是创建一个EXCEL,并在主函数中将想要写入的字符串写入对应的EXCEL的行列中去
时间: 2024-04-10 15:33:08 浏览: 15
当然可以!下面是一个示例代码,可以在VS2017中使用C#创建一个类,并在主函数中将字符串写入Excel的行列中:
```csharp
using System;
using System.IO;
using Excel = Microsoft.Office.Interop.Excel;
namespace ExcelWriter
{
class ExcelCreator
{
public void CreateAndWriteToExcel(string filePath, string[,] data)
{
// 创建Excel应用程序对象
Excel.Application excelApp = new Excel.Application();
excelApp.Visible = true;
// 添加一个工作簿
Excel.Workbook workbook = excelApp.Workbooks.Add();
// 获取第一个工作表
Excel.Worksheet worksheet = (Excel.Worksheet)workbook.Sheets[1];
// 将数据写入Excel的行列中
int rows = data.GetLength(0);
int columns = data.GetLength(1);
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < columns; j++)
{
worksheet.Cells[i + 1, j + 1] = data[i, j];
}
}
// 保存Excel文件
workbook.SaveAs(filePath);
// 关闭工作簿和Excel应用程序对象
workbook.Close();
excelApp.Quit();
// 释放COM对象
System.Runtime.InteropServices.Marshal.ReleaseComObject(worksheet);
System.Runtime.InteropServices.Marshal.ReleaseComObject(workbook);
System.Runtime.InteropServices.Marshal.ReleaseComObject(excelApp);
GC.Collect(); // 回收垃圾对象
}
}
class Program
{
static void Main(string[] args)
{
string filePath = "C:\\path\\to\\your\\excel.xlsx";
string[,] data = new string[,]
{
{ "A1", "B1", "C1" },
{ "A2", "B2", "C2" },
{ "A3", "B3", "C3" }
};
ExcelCreator excelCreator = new ExcelCreator();
excelCreator.CreateAndWriteToExcel(filePath, data);
Console.WriteLine("数据已写入Excel文件!");
Console.ReadLine();
}
}
}
```
请确保在运行代码前,已将 `Microsoft.Office.Interop.Excel` 程序集添加到项目引用中。此外,还需根据实际情况修改 `filePath` 和 `data` 的值。
希望对你有所帮助!如果有任何问题,请随时提问。
相关推荐
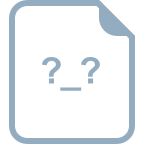
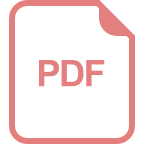














