判断玩家“石头剪刀布”游戏输赢的C语言程序
时间: 2024-09-11 12:13:43 浏览: 26
在C语言中编写一个简单的"石头、剪刀、布"游戏的程序,可以包括用户输入判断部分和随机选择部分。以下是一个基本的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h> // 引入时间库用于生成随机数
// 定义石头、剪刀、布的常量
enum Gesture { ROCK = 0, SCISSORS = 1, PAPER = 2 };
int get_user_choice() {
int choice;
printf("请输入(0 石头, 1 剪刀, 2 布): ");
scanf("%d", &choice);
return choice;
}
int computer_choice() {
srand(time(NULL)); // 初始化随机数种子
return rand() % 3; // 生成0到2的随机整数代表石头、剪刀或布
}
void determine_winner(enum Gesture user, enum Gesture computer) {
if (user == computer) {
printf("平局!\n");
} else if ((user == ROCK && computer == SCISSORS) ||
(user == SCISSORS && computer == PAPER) ||
(user == PAPER && computer == ROCK)) {
printf("你赢了!\n");
} else {
printf("电脑赢了!它出了 %s。\n", gesture_to_string[computer]);
}
}
char* gesture_to_string[] = {"石头", "剪刀", "布"};
int main() {
enum Gesture user = get_user_choice();
enum Gesture computer = computer_choice();
determine_winner(user, computer);
return 0;
}
```
在这个程序中,用户通过键盘输入他们的选择,而电脑则是通过随机数生成器来出拳。`determine_winner`函数比较了用户的输入和电脑的选择,然后输出结果。
相关推荐
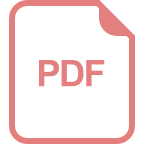
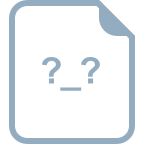
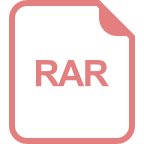
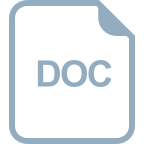

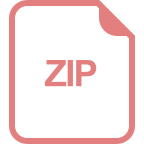
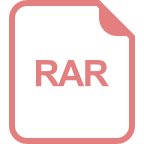
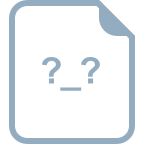
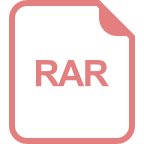
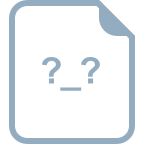







