httpclient发送post请求 带文件与参数
时间: 2023-12-08 17:07:03 浏览: 217
要使用HttpClient发送带文件和参数的POST请求,您可以按照以下步骤进行操作:
1. 导入所需的类:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.entity.mime.content.StringBody;
import org.apache.http.impl.client.HttpClients;
```
2. 创建HttpClient实例:
```java
HttpClient httpClient = HttpClients.createDefault();
```
3. 创建HttpPost请求对象:
```java
HttpPost httpPost = new HttpPost(url);
```
其中,`url`是目标URL。
4. 创建MultipartEntityBuilder对象,并添加文件和参数:
```java
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addPart("file", new FileBody(file)); // 添加文件
builder.addPart("param1", new StringBody(param1, ContentType.TEXT_PLAIN)); // 添加参数1
builder.addPart("param2", new StringBody(param2, ContentType.TEXT_PLAIN)); // 添加参数2
```
其中,`file`是要上传的文件,`param1`和`param2`是需要传递的参数。
5. 构建HttpEntity并设置给HttpPost请求对象:
```java
HttpEntity multipartEntity = builder.build();
httpPost.setEntity(multipartEntity);
```
6. 执行HttpPost请求并获取响应:
```java
HttpResponse response = httpClient.execute(httpPost);
```
7. 处理响应结果:
```java
int statusCode = response.getStatusLine().getStatusCode();
HttpEntity responseEntity = response.getEntity();
// 处理响应实体
```
完整的示例代码如下:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.entity.mime.content.StringBody;
import org.apache.http.impl.client.HttpClients;
import java.io.File;
import java.io.IOException;
public class HttpClientExample {
public static void main(String[] args) {
String url = "http://example.com/upload";
String filePath = "/path/to/file";
String param1 = "value1";
String param2 = "value2";
HttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addPart("file", new FileBody(new File(filePath)));
builder.addPart("param1", new StringBody(param1, ContentType.TEXT_PLAIN));
builder.addPart("param2", new StringBody(param2, ContentType.TEXT_PLAIN));
HttpEntity multipartEntity = builder.build();
httpPost.setEntity(multipartEntity);
try {
HttpResponse response = httpClient.execute(httpPost);
int statusCode = response.getStatusLine().getStatusCode();
HttpEntity responseEntity = response.getEntity();
// 处理响应实体
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请注意,上述示例中使用的是Apache HttpClient 4.x版本。在使用之前,请确保已经添加相关的依赖。
阅读全文
相关推荐
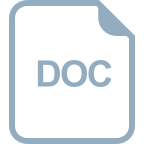
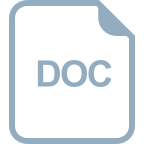
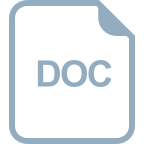
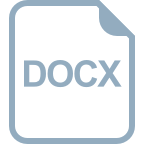
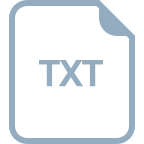
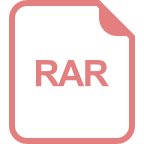
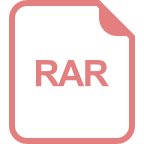
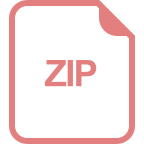
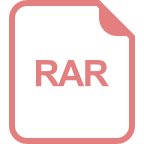
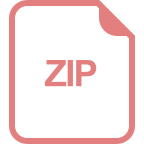





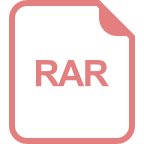


