vue 手写日历排班
时间: 2023-10-24 09:10:12 浏览: 58
好的,我可以为您提供一些思路。首先,您需要确定日历的展示方式,是按周展示还是按月展示。然后,您需要确定排班的数据结构,比如使用一个二维数组来表示每个日期的排班情况。最后,您需要实现一个算法来根据排班数据生成日历。
以下是一个简单的实现思路:
1. 确定日历展示方式:按月展示。
2. 确定排班数据结构:使用一个二维数组来表示每个日期的排班情况,数组的每个元素表示一个日期,包含日期、星期几、是否工作日、是否休息日、是否有排班等信息。
3. 实现算法:根据排班数据生成日历。具体实现可以参考以下步骤:
- 根据当前年月计算出该月的天数。
- 遍历每一天,根据排班数据填充每一天的信息。
- 根据填充好的信息生成日历。
以下是一个简单的代码实现:
```javascript
<template>
<div>
<table>
<thead>
<tr>
<th v-for="day in days">{{ day }}</th>
</tr>
</thead>
<tbody>
<tr v-for="week in weeks">
<td v-for="day in week" :class="{ 'workday': day.workday, 'restday': day.restday }">
{{ day.date }}
</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
days: ['日', '一', '二', '三', '四', '五', '六'],
weeks: [],
schedule: [
{ date: 1, workday: true },
{ date: 2, workday: true },
{ date: 3, workday: true },
{ date: 4, workday: true },
{ date: 5, workday: true },
{ date: 6, restday: true },
{ date: 7, restday: true },
{ date: 8, workday: true },
{ date: 9, workday: true },
{ date: 10, workday: true },
{ date: 11, workday: true },
{ date: 12, workday: true },
{ date: 13, restday: true },
{ date: 14, restday: true },
{ date: 15, workday: true },
{ date: 16, workday: true },
{ date: 17, workday: true },
{ date: 18, workday: true },
{ date: 19, workday: true },
{ date: 20, restday: true },
{ date: 21, restday: true },
{ date: 22, workday: true },
{ date: 23, workday: true },
{ date: 24, workday: true },
{ date: 25, workday: true },
{ date: 26, workday: true },
{ date: 27, restday: true },
{ date: 28, restday: true },
{ date: 29, workday: true },
{ date: 30, workday: true },
{ date: 31, workday: true },
],
};
},
mounted() {
this.generateCalendar();
},
methods: {
generateCalendar() {
const firstDay = new Date(new Date().getFullYear(), new Date().getMonth(), 1).getDay();
const lastDate = new Date(new Date().getFullYear(), new Date().getMonth() + 1, 0).getDate();
let date = 1;
for (let i = 0; i < 6; i++) {
const week = [];
for (let j = 0; j < 7; j++) {
if (i === 0 && j < firstDay) {
week.push({});
} else if (date > lastDate) {
week.push({});
} else {
const day = this.schedule.find((item) => item.date === date);
week.push(day || { date });
date++;
}
}
this.weeks.push(week);
}
},
},
};
</script>
<style>
.workday {
background-color: #fff;
}
.restday {
background-color: #eee;
}
</style>
```
相关推荐
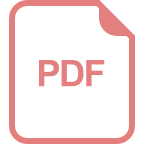














