麻雀搜索算法的实际应用附带代码
时间: 2024-11-19 22:18:32 浏览: 5
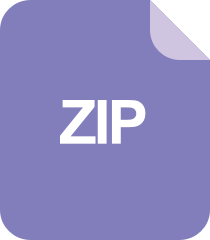
麻雀搜索算法优化支持向量机python

麻雀搜索算法(Pigeon Search Optimization,PSO),也被称为鸽巢优化或信鸽寻优算法,是一种启发式搜索算法,它模仿了鸟群的行为模式,尤其是信鸽返回窝的过程。PSO主要用于解决复杂的优化问题,如函数最小化、路径规划等。
实际应用中,PSO常用于以下几个领域:
1. **工程设计**:例如在机械、电子或材料等领域寻找最优设计方案。
2. **机器学习**:作为特征选择或超参数优化的一部分。
3. **数据挖掘**:优化模型的参数设置以提高预测性能。
以下是简单的Python代码示例(使用`scipy.optimize`库实现了PSO):
```python
import numpy as np
from scipy.optimize import minimize
# 定义简单的一维函数作为优化目标
def func(x):
return x**2 - 2*x + 5
# 初始化鸽巢搜索算法
def pso(func, bounds, n_particles=20, max_iter=100):
# 初始化粒子位置和速度
particles = np.random.uniform(bounds[:, 0], bounds[:, 1], (n_particles, len(bounds)))
velocities = np.zeros_like(particles)
best_positions = particles.copy()
gbest_position = min(best_positions, key=func)
for _ in range(max_iter):
# 更新粒子速度
r1, r2 = np.random.rand(n_particles, len(bounds)), np.random.rand(n_particles, len(bounds))
velocities = r1 * c1 * (gbest_position - particles) + r2 * c2 * (particles - best_positions)
# 更新粒子位置
particles += velocities
# 确保粒子位于边界范围内
particles = np.clip(particles, bounds[:, 0], bounds[:, 1])
# 更新局部最佳和全局最佳
updated_best_positions = particles[np.argsort(func(particles))[:n_particles]]
gbest_position = min(updated_best_positions, key=func)
return gbest_position, func(gbest_position)
# 示例参数
bounds = [(0, 5), (0, 5)] # 可能的解空间范围
c1, c2 = 2.0, 2.0 # 学习因子
result = pso(func, bounds)
print("全局最佳解:", result[0], "对应的函数值:", result[1])
阅读全文
相关推荐
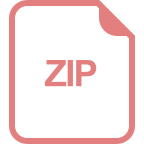
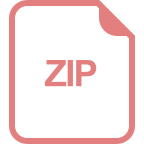
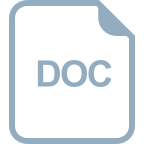
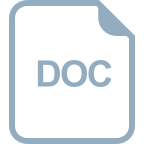
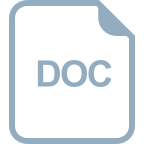
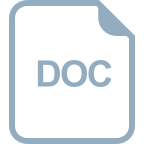
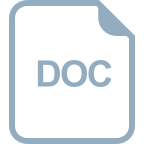
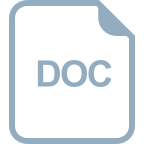
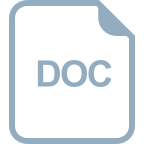
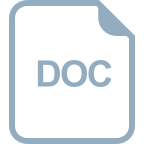
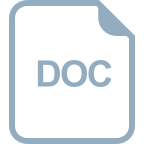
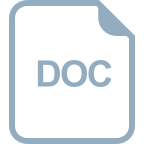
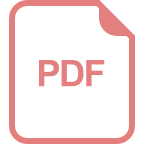
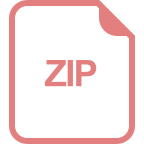
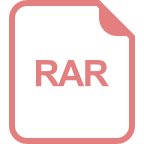
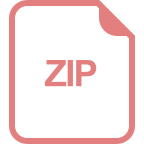
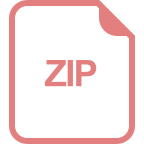
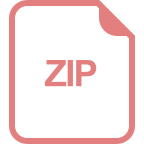