用c语言写一个简单的程序,定义一个结构体数组,输入结构体数组元素,并编写函数对结构体数组中插 入记录;
时间: 2024-08-13 14:07:23 浏览: 69
当你想要在C语言中定义一个结构体数组并插入新的记录时,首先你需要定义一个结构体,然后定义数组并为用户提供输入的接口。这里是一个简单的例子:
```c
#include <stdio.h>
#include <string.h>
// 定义一个结构体
struct Student {
char name[50];
int age;
};
// 定义插入新记录的函数
void insertRecord(struct Student arr[], int *size, char* name, int age) {
if (*size >= sizeof(arr) / sizeof(arr)) {
printf("Array is full, cannot insert more records.\n");
return;
}
strcpy(arr[*size].name, name);
arr[*size].age = age;
(*size)++;
printf("Record inserted successfully.\n");
}
int main() {
const int MAX_SIZE = 10; // 结构体数组的最大大小
struct Student students[MAX_SIZE]; // 定义结构体数组
int size = 0; // 记录数组的实际大小
// 输入结构体数组元素
while (size < MAX_SIZE) {
char name[50];
int age;
printf("Enter name and age for student %d: ", size + 1);
scanf("%s%d", name, &age);
insertRecord(students, &size, name, age);
}
// 打印整个数组的内容
printf("\nStudents in the array:\n");
for (int i = 0; i < size; i++) {
printf("Name: %s, Age: %d\n", students[i].name, students[i].age);
}
return 0;
}
```
在这个例子中,我们定义了一个`Student`结构体,包含名字和年龄两个字段。`insertRecord`函数接收结构体数组、数组当前大小指针、新记录的名字和年龄。在`main`函数中,我们允许用户输入结构体数组的元素,直到达到最大容量。最后,我们打印出整个数组的内容。
阅读全文
相关推荐
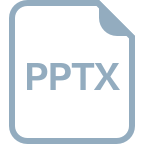
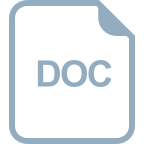
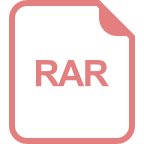





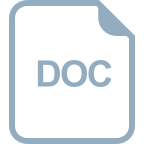
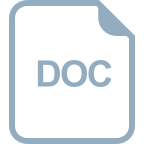
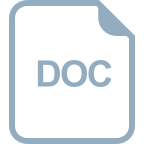
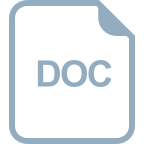






