实现一个简单的计算器编写一个C程序,实现一个能够处理多位数运算、括号优先级和多种运算符(如加、减、乘、除、取余、乘方等)的计算器。
时间: 2024-12-21 19:24:42 浏览: 3
为了实现一个简单的计算器,你可以使用C语言中的基本控制结构(如循环、条件判断),以及栈数据结构来处理运算符的优先级和括号。下面是一个简化的示例程序,它使用了递归下降解析器的方式来实现这个功能。注意,这只是一个基础版本,实际应用可能需要更完善的错误处理和输入验证。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_TOKEN_LEN 50
#define OPERATORS "+-*/%^"
typedef struct {
char operator;
int precedence;
} Operator;
Operator operators[] = {
{'+', 1},
{'-', 1},
{'*', 2},
{'/', 2},
{'%', 2},
{'^', 3}
};
char* tokenize(const char *input);
double apply_operator(double a, double b, char op);
int main() {
char input[1000];
printf("Enter an expression: ");
fgets(input, sizeof(input), stdin);
input[strcspn(input, "\n")] = '\0'; // Remove newline character
char *tokens = tokenize(input);
double result = evaluate(tokens);
printf("Result: %.2lf\n", result);
free(tokens);
return 0;
}
char* tokenize(const char *input) {
char *tokens = malloc(sizeof(char*) * 10);
int i = 0, j = 0;
while (input[j] != '\0') {
if (isdigit(input[j])) {
tokens[i++] = input + j;
while (j < strlen(input) && isdigit(input[j + 1])) {
j++;
}
tokens[i++] = '\0';
} else if (input[j] == ' ' || input[j] == '\t' || input[j] == '\n') {
continue;
} else if (strchr(OPERATORS, input[j]) != NULL) {
tokens[i++] = input + j;
j++;
} else {
break;
}
}
tokens[i] = '\0'; // Terminate the array
return tokens;
}
double evaluate(char** tokens) {
double num1 = atof(tokens[0]);
for (int i = 1; tokens[i]; i += 2) { // Skip every two elements as they're pairs of number and operator
double num2 = atof(tokens[i]);
char op = tokens[i+1][0];
num1 = apply_operator(num1, num2, op);
}
return num1;
}
double apply_operator(double a, double b, char op) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
if (b != 0)
return a / b;
else
return 0; // Handle division by zero
case '%':
if (b != 0)
return fmod(a, b);
else
return 0; // Handle modulo by zero
case '^':
return pow(a, b);
default:
return 0; // Invalid operator
}
}
```
阅读全文
相关推荐
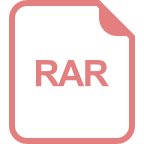
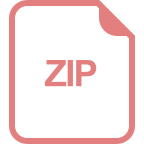
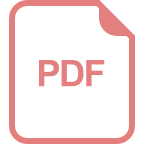
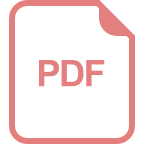
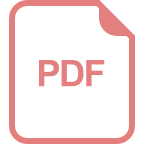
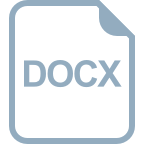
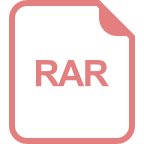
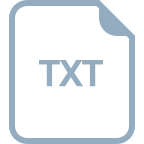
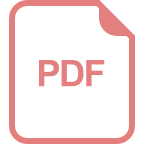
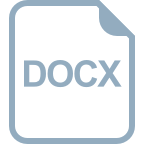
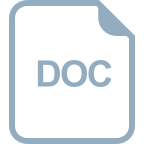
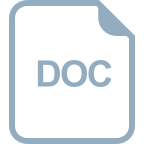
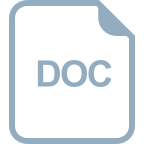
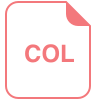
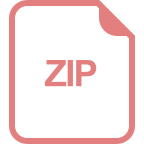