用java写一个饮品店点单系统
时间: 2023-12-31 11:05:12 浏览: 98
这是一个简单的饮品店点单系统,使用Java编写,可以选择饮品种类、数量和加料,并计算总价格。
```java
import java.util.Scanner;
public class DrinkShop {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 饮品种类
String[] drinks = {"Bubble Milk Tea", "Green Tea", "Black Tea", "Milk Coffee"};
// 饮品价格
int[] prices = {20, 15, 15, 25};
// 加料种类
String[] toppings = {"Pearls", "Pudding", "Grass Jelly", "Coconut Jelly"};
// 加料价格
int[] toppingPrices = {5, 10, 8, 10};
System.out.println("Welcome to Drink Shop!");
// 选择饮品
System.out.println("Please select a drink:");
for (int i = 0; i < drinks.length; i++) {
System.out.println((i + 1) + ". " + drinks[i] + " (" + prices[i] + " yuan)");
}
int drinkIndex = scanner.nextInt() - 1;
System.out.println("You have selected " + drinks[drinkIndex] + ".");
// 选择数量
System.out.println("How many cups do you want?");
int quantity = scanner.nextInt();
System.out.println("You want " + quantity + " cups of " + drinks[drinkIndex] + ".");
// 选择加料
System.out.println("Do you want to add toppings? (y/n)");
String choice = scanner.next();
int toppingPrice = 0;
if (choice.equalsIgnoreCase("y")) {
System.out.println("Please select a topping:");
for (int i = 0; i < toppings.length; i++) {
System.out.println((i + 1) + ". " + toppings[i] + " (" + toppingPrices[i] + " yuan)");
}
int toppingIndex = scanner.nextInt() - 1;
System.out.println("You have selected " + toppings[toppingIndex] + ".");
toppingPrice = toppingPrices[toppingIndex];
}
// 计算总价格
int totalPrice = prices[drinkIndex] * quantity + toppingPrice * quantity;
System.out.println("Your total price is " + totalPrice + " yuan.");
System.out.println("Thank you for shopping at Drink Shop!");
}
}
```
阅读全文
相关推荐








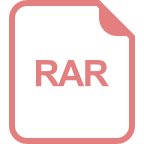






