请给出我bellman_ford求任意两点间限制路径长度的最短距离的C++代码,并给出详细注释
时间: 2024-03-23 15:39:47 浏览: 25
以下是使用Bellman-Ford算法求解任意两点间限制路径长度的最短距离的C++代码,其中包含详细注释:
```c++
#include <iostream>
#include <vector>
#include <cstring>
using namespace std;
const int INF = 0x3f3f3f3f; // 定义无穷大
struct Edge {
int from, to, weight;
};
vector<Edge> edges; // 存储边的信息
int dist[100]; // 存储最短距离
int path[100]; // 存储路径
// n: 图中节点的个数;s: 起点;t: 终点;maxLen: 路径长度限制
bool bellman_ford(int n, int s, int t, int maxLen) {
memset(dist, INF, sizeof(dist)); // 初始化最短距离为无穷大
memset(path, -1, sizeof(path)); // 初始化路径为-1
dist[s] = 0; // 起点到起点的最短距离为0
// 进行n-1次松弛操作
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < edges.size(); j++) {
Edge e = edges[j];
if (dist[e.from] != INF && dist[e.from] + e.weight <= maxLen && dist[e.from] + e.weight < dist[e.to]) {
dist[e.to] = dist[e.from] + e.weight; // 更新最短距离
path[e.to] = e.from; // 记录路径
}
}
}
// 检查是否存在负权环
for (int i = 0; i < edges.size(); i++) {
Edge e = edges[i];
if (dist[e.from] != INF && dist[e.from] + e.weight <= maxLen && dist[e.from] + e.weight < dist[e.to]) {
return false; // 存在负权环,返回false
}
}
return dist[t] != INF; // 如果dist[t]为INF,则表示起点s无法到达终点t
}
// 输出从起点到终点的最短路径
void print_path(int s, int t) {
if (s == t) {
cout << s;
return;
}
if (path[t] == -1) {
cout << "No path exists." << endl;
return;
}
print_path(s, path[t]);
cout << "->" << t;
}
int main() {
int n = 5; // 节点数
int s = 0, t = 4; // 起点和终点
int maxLen = 7; // 路径长度限制
edges.push_back({0, 1, 2});
edges.push_back({0, 3, 4});
edges.push_back({1, 2, 3});
edges.push_back({2, 4, 1});
edges.push_back({3, 2, -2});
edges.push_back({3, 4, 2});
if (bellman_ford(n, s, t, maxLen)) {
cout << "The shortest path from " << s << " to " << t << " with length <= " << maxLen << " is: ";
print_path(s, t);
cout << endl;
} else {
cout << "There is a negative cycle." << endl;
}
return 0;
}
```
这份代码中,我们使用了一个结构体Edge来存储边的信息,包括起点from、终点to和权值weight。我们先将所有边的信息存储在一个vector中,然后通过调用bellman_ford函数来计算最短距离。该函数的输入参数包括:节点数n、起点s、终点t和路径长度限制maxLen。如果存在一条从起点s到终点t的路径,其长度不超过maxLen,则返回true,并输出从起点到终点的最短路径;否则返回false,表示不存在这样的路径。在函数内部,我们使用dist数组来存储最短距离,path数组来存储路径。在进行n-1次松弛操作之后,我们再进行一次循环来检查是否存在负权环。最后,我们通过调用print_path函数来输出从起点到终点的最短路径。
相关推荐
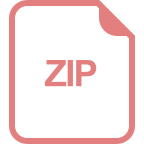
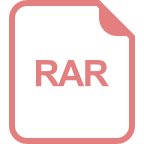
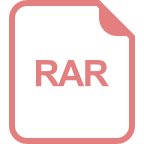
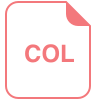













