编写一段python代码,计算PSO-kmeans的SSE
时间: 2024-03-02 09:25:23 浏览: 85
以下是计算PSO-Kmeans的SSE的Python代码:
```python
import numpy as np
from sklearn.cluster import KMeans
def pso_kmeans_sse(X, k, w=0.5, c1=0.8, c2=0.9, max_iter=100):
"""
Calculate the sum of squared errors (SSE) for PSO-Kmeans clustering algorithm
Params:
- X: ndarray, shape (n_samples, n_features), input data for clustering
- k: int, number of clusters to group the data
- w: float, inertia weight to balance exploration and exploitation, default 0.5
- c1: float, cognitive parameter to control the effect of personal best, default 0.8
- c2: float, social parameter to control the effect of global best, default 0.9
- max_iter: int, maximum number of iterations to run the algorithm, default 100
Returns:
- sse: float, the sum of squared errors for the final clustering result
"""
# Initialize particles
n_samples = X.shape[0]
particles = np.random.rand(k, n_samples, X.shape[1])
velocities = np.zeros((k, n_samples, X.shape[1]))
p_best_pos = particles.copy()
p_best_score = np.ones(k) * np.inf
g_best_pos = None
g_best_score = np.inf
# Run PSO-Kmeans algorithm
for _ in range(max_iter):
# Update particles' locations and velocities
for i in range(k):
for j in range(n_samples):
# Update velocity
r1 = np.random.rand()
r2 = np.random.rand()
velocities[i, j] = w * velocities[i, j] \
+ c1 * r1 * (p_best_pos[i, j] - particles[i, j]) \
+ c2 * r2 * (g_best_pos[i] - particles[i, j])
# Update position
particles[i, j] = particles[i, j] + velocities[i,j]
# Calculate the fitness score for each particle and update personal bests
for i in range(k):
kmeans = KMeans(n_clusters=k, init=particles[i])
kmeans.fit(X)
sse = kmeans.inertia_
if sse < p_best_score[i]:
p_best_score[i] = sse
p_best_pos[i] = particles[i].copy()
# Update global best if necessary
if sse < g_best_score:
g_best_score = sse
g_best_pos = particles[i].copy()
return g_best_score
```
上述代码中使用了sklearn中的KMeans方法,通过PSO-Kmeans来进行严格数值上的聚类,计算出最终的SSE值。
阅读全文
相关推荐
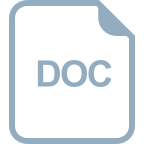
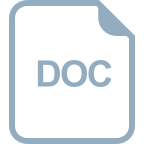
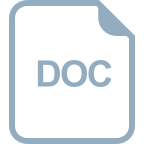
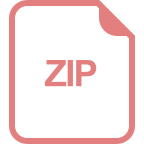


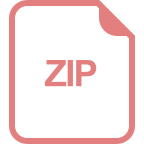
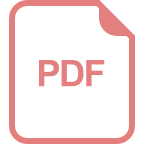
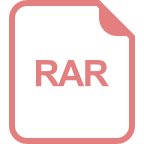
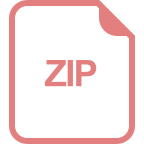
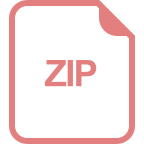
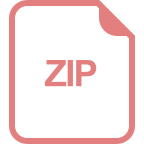
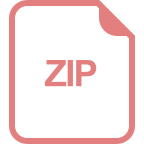
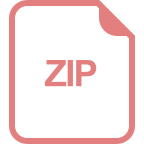
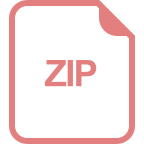
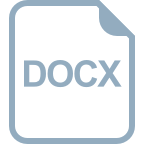
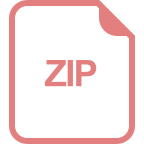
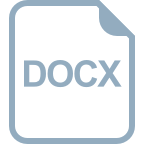
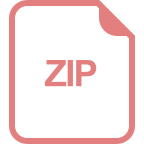