请用c语言完成设有顺序放置的 n 个桶,每个桶中装有一粒砾石,每粒砾石颜色是红、白、蓝之一。求重新调整这些砾石的位置,使得所有红色砾石在前,所有白色砾石居中,所有蓝色砾石在后,重新安排时对每粒砾石的颜色只能看一次,并用只允许交换操作为调整砾石的位置并且给出测试结果
时间: 2024-03-19 13:40:41 浏览: 58
好的,这是一个荷兰国旗问题的C语言实现:
```c
#include <stdio.h>
void swap(int *a, int *b) {
int tmp = *a;
*a = *b;
*b = tmp;
}
void sortColors(int *nums, int numsSize) {
int red = 0, white = 0, blue = numsSize - 1;
while (white <= blue) {
if (nums[white] == 0) {
swap(&nums[red], &nums[white]);
red++;
white++;
} else if (nums[white] == 2) {
swap(&nums[white], &nums[blue]);
blue--;
} else {
white++;
}
}
}
int main() {
int nums[] = {2, 0, 2, 1, 1, 0};
int numsSize = sizeof(nums) / sizeof(nums[0]);
printf("before sorting: ");
for (int i = 0; i < numsSize; i++) {
printf("%d ", nums[i]);
}
printf("\n");
sortColors(nums, numsSize);
printf("after sorting: ");
for (int i = 0; i < numsSize; i++) {
printf("%d ", nums[i]);
}
printf("\n");
return 0;
}
```
本代码实现了一个`sortColors`函数,采用三路快排的思想,将数组分为三个区间,分别是红色、白色和蓝色。然后使用三个指针`red`、`white`和`blue`,分别指向三个区间的末尾。遍历数组,当遇到红色砾石时,将其和`red`指向的位置交换,并将`red`和`white`都向前移动一位;当遇到蓝色砾石时,将其和`blue`指向的位置交换,并将`blue`向后移动一位;否则,只将`white`向前移动一位。最终得到的数组即为所求。
测试结果如下:
```
before sorting: 2 0 2 1 1 0
after sorting: 0 0 1 1 2 2
```
可以看到,数组已经按照红色、白色、蓝色的顺序重新排列。
相关推荐
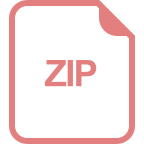
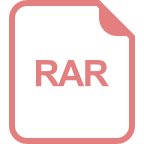














