用C写一个往sqlite数据库插入数据库的demo
时间: 2024-05-14 19:13:18 浏览: 18
Sure, here's a basic demo of inserting data into an SQLite database using C:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sqlite3.h>
int main()
{
sqlite3 *db;
char *err_msg = 0;
int rc = sqlite3_open("mydatabase.db", &db);
if (rc != SQLITE_OK) {
fprintf(stderr, "Cannot open database: %s\n", sqlite3_errmsg(db));
sqlite3_close(db);
return 1;
}
char *sql = "INSERT INTO users (name, age, email) VALUES ('John', 25, 'john@example.com')";
rc = sqlite3_exec(db, sql, 0, 0, &err_msg);
if (rc != SQLITE_OK ) {
fprintf(stderr, "SQL error: %s\n", err_msg);
sqlite3_free(err_msg);
return 1;
}
sqlite3_close(db);
return 0;
}
```
This code assumes that you have a database named `mydatabase.db` and a table named `users` with columns `name`, `age`, and `email`. It inserts a new row into the table with values of `'John'`, `25`, and `'john@example.com'`. Of course, in a real-world scenario, you would probably want to use parameters instead of hard-coding the values in the SQL statement.
相关推荐
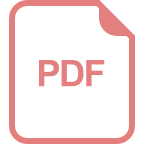
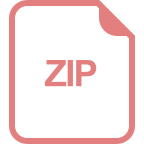
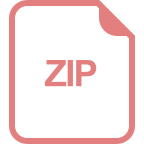









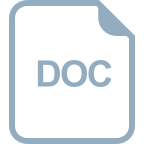
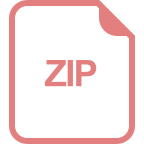