ds3231 century
时间: 2023-10-29 15:07:08 浏览: 147
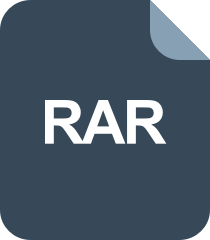
Arduino--DS3231时钟模块
DS3231芯片中并没有直接存储世纪信息,而是使用了一个称为“年份”的寄存器来存储年份信息。其中,年份信息的高位表示世纪信息,低位表示年份信息。世纪信息为0表示公元2000年至2099年,为1表示公元2100年至2199年,以此类推。
如果您想从DS3231芯片中读取年份信息,可以使用Wire库发送读取命令,并使用DS3231库中的getYear()函数来获取年份信息,如下所示:
```
#include <Wire.h>
#include "RTClib.h"
RTC_DS3231 rtc;
void setup()
{
Wire.begin();
rtc.begin();
// 获取年份信息
uint16_t year = rtc.getYear();
// 计算世纪信息
uint8_t century = year / 100;
// 打印世纪信息
Serial.print("Century: ");
Serial.println(century);
}
void loop()
{
// 等待一段时间
delay(1000);
}
```
在上面的代码中,我们使用Wire库初始化I2C通信,并使用RTC_DS3231库初始化DS3231芯片。然后,我们使用getYear()函数获取年份信息,并计算出世纪信息。最后,我们使用Serial.println()函数将世纪信息打印到串口监视器中。
阅读全文
相关推荐
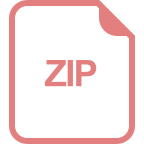
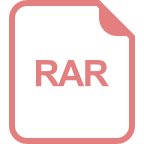
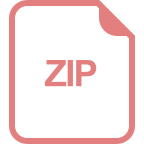
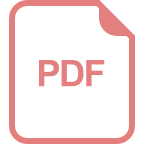
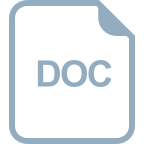
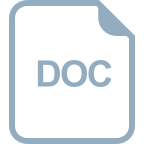

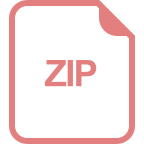
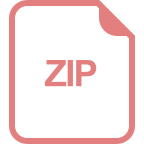
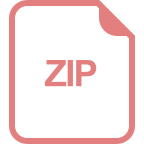
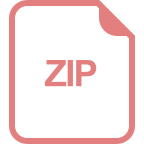
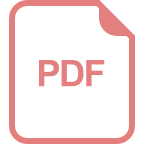
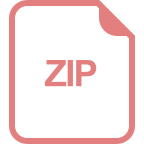