Gatling CoAP插件示例
时间: 2023-07-08 19:35:16 浏览: 147
以下是一个简单的Gatling CoAP插件示例:
```scala
package com.example.gatling.coap
import akka.actor.ActorSystem
import akka.stream.ActorMaterializer
import io.gatling.commons.validation._
import io.gatling.core.action.builder.ActionBuilder
import io.gatling.core.session.Session
import io.gatling.core.structure.ScenarioContext
import io.gatling.core.util.NameGen
import org.eclipse.californium.core.coap.{CoAP, Request, Response}
import org.eclipse.californium.core.network.{CoapEndpoint, EndpointContext}
import scala.concurrent.Future
import scala.util.{Failure, Success}
/**
* A Gatling CoAP action that sends a CoAP request and waits for the response.
*/
class CoapAction(requestName: String, request: Request, endpoint: CoapEndpoint)(implicit system: ActorSystem, materializer: ActorMaterializer)
extends ActionBuilder with NameGen {
override def build(ctx: ScenarioContext, next: ActionBuilder): akka.actor.ActorRef = {
import ctx.{coreComponents, protocolComponentsRegistry}
val coapComponents = protocolComponentsRegistry.components(CoapProtocol.CoapProtocolKey)
coreComponents.actorSystem.actorOf(CoapActionActor.props(requestName, request, endpoint, coapComponents.coapClient), genName("coapRequest"))
}
private class CoapActionActor(requestName: String, request: Request, endpoint: CoapEndpoint, coapClient: CoapClient) extends akka.actor.Actor with NameGen {
implicit val ec = context.dispatcher
override def receive: Receive = {
case session: Session =>
val outcome = sendRequest(request, endpoint, coapClient)
.map(response => processResponse(session, response))
.recover {
case e: Exception => session.markAsFailed(Some(e.getMessage)).failure
}
outcome.onComplete(result => context.parent ! result)
}
private def sendRequest(request: Request, endpoint: CoapEndpoint, coapClient: CoapClient): Future[Response] = {
val promise = scala.concurrent.Promise[Response]()
request.addMessageObserver(new org.eclipse.californium.core.coap.MessageObserver {
override def onAcknowledgement(): Unit = {}
override def onReject(): Unit = {}
override def onResponse(response: Response): Unit = {
promise.success(response)
}
override def onTimeout(): Unit = {
promise.failure(new Exception("Request timed out"))
}
})
coapClient.sendRequest(request, endpoint, new EndpointContext())
promise.future
}
private def processResponse(session: Session, response: Response): Validation[Unit] = {
val statusCode = response.getCode.value
if (statusCode >= CoAP.ResponseCode.BAD_REQUEST.value) {
session.markAsFailed(Some(s"CoAP request failed with status $statusCode")).failure
} else {
session.markAsSucceeded.success
}
}
}
}
```
该插件包含一个CoapAction类,该类负责发送CoAP请求和等待响应。该类使用了Eclipse Californium库来发送和接收CoAP消息。在发送请求之后,CoapActionActor类将等待响应,并将响应发送回父Actor,以便Gatling可以将其与其他请求进行协调。
为了使用该插件,您需要在测试中创建一个CoapProtocol实例,并将其传递给Gatling的setUp方法,例如:
```scala
import io.gatling.core.Predef._
import io.gatling.coap.Predef._
import org.eclipse.californium.core.coap.{CoAP, Request}
class MyScenario extends Simulation {
val coapProtocol = coap("coap://localhost:5683")
val request = new Request(CoAP.Code.GET)
request.setURI("/hello")
val scn = scenario("CoAP request")
.exec(coap(requestName = "getHello", request = request, endpoint = coapProtocol.endpoint))
setUp(scn.inject(atOnceUsers(1))).protocols(coapProtocol)
}
```
在这个示例中,我们创建了一个CoAP请求,并使用coap方法创建了一个CoapProtocol实例。我们还创建了一个场景,并在其中执行了一个CoapAction,该动作将发送我们的请求并等待响应。最后,我们使用setUp方法将场景和CoapProtocol实例传递给Gatling,以便它可以运行我们的测试。
阅读全文
相关推荐
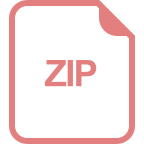
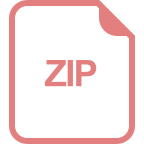
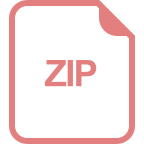
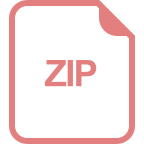
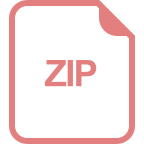
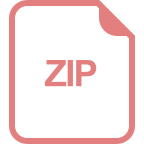
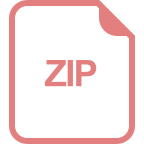
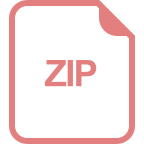
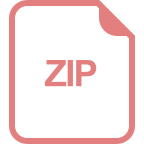
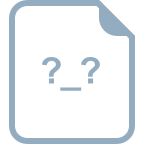
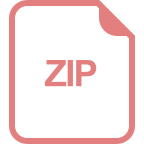
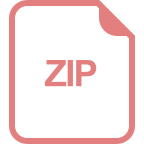
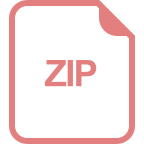
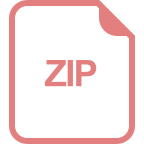
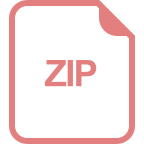
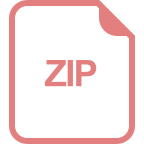
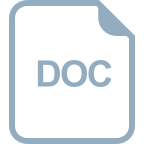


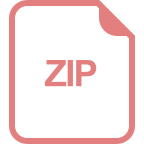