如何查看springboot项目中的全部线程池信息
时间: 2023-09-03 15:04:54 浏览: 157
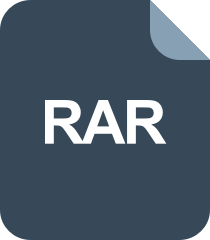
Java开发案例-springboot-60-整合DynamicTp动态线程池-源代码+文档.rar
### 回答1:
如果你想查看 Spring Boot 项目中的全部线程池信息,你可以使用下列方法之一:
1. 使用 Spring Boot Actuator:Spring Boot Actuator 是 Spring Boot 的一个模块,可以提供对应用程序内部状态的访问。可以通过访问 Actuator 端点来查看线程池信息。你可以在 `application.properties` 文件中配置 Actuator 端点,然后在浏览器中访问对应的 URL 来查看线程池信息。例如,你可以在 `application.properties` 中添加以下配置:
```
management.endpoints.web.exposure.include=*
```
然后,你可以在浏览器中访问 `http://localhost:8080/actuator/threadpools` 来查看线程池信息。
2. 使用 JMX:可以使用 JMX(Java Management Extension)来查看线程池信息。你需要在 `application.properties` 中配置 JMX,然后使用 JMX 工具(例如 jconsole)连接到应用程序并查看线程池信息。你可以在 `application.properties` 中添加以下配置启用 JMX:
```
spring.jmx.enabled=true
```
然后,你可以使用 jconsole 连接到应用程序,并在 MBeans 选项卡中查看线程池信息。
3. 使用日志:你可以在应用程序中使用日志打印线程池信息。例如,你可以在代码中使用 `ThreadPool
### 回答2:
要查看Spring Boot项目中的全部线程池信息,可以使用Java Management Extensions (JMX)来监视和管理应用程序的各种资源,包括线程池。
首先,在Spring Boot项目的配置文件中加入以下配置,开启JMX监视和管理功能:
```
spring.jmx.enabled=true
```
然后,在Spring Boot项目的源代码中添加以下代码,用于暴露线程池的信息:
```java
import org.springframework.boot.actuate.endpoint.annotation.Endpoint;
import org.springframework.boot.actuate.endpoint.annotation.ReadOperation;
import org.springframework.stereotype.Component;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.ThreadPoolExecutor;
@Component
@Endpoint(id = "threadpools")
public class ThreadPoolEndpoint {
private final Map<String, ThreadPoolExecutor> threadPools = new HashMap<>();
public void registerThreadPool(String name, ThreadPoolExecutor executor) {
threadPools.put(name, executor);
}
@ReadOperation
public Map<String, Object> threadPools() {
Map<String, Object> result = new HashMap<>();
for (Map.Entry<String, ThreadPoolExecutor> entry : threadPools.entrySet()) {
Map<String, Object> threadPoolInfo = new HashMap<>();
ThreadPoolExecutor executor = entry.getValue();
threadPoolInfo.put("activeThreads", executor.getActiveCount());
threadPoolInfo.put("completedTasks", executor.getCompletedTaskCount());
// 可以根据需要添加更多的线程池信息
result.put(entry.getKey(), threadPoolInfo);
}
return result;
}
}
```
以上代码定义了一个自定义的Endpoint,用于暴露线程池信息。通过`registerThreadPool`方法,将线程池的实例注册到Endpoint中。通过`threadPools`方法,可以获取到所有线程池的信息。
最后,在Spring Boot项目启动类中,将自定义的Endpoint注入到管理端点中:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class Application {
@Autowired
private ThreadPoolEndpoint threadPoolEndpoint;
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
public ThreadPoolEndpoint threadPoolEndpoint() {
return new ThreadPoolEndpoint();
}
@PostConstruct
public void init() {
// 注册线程池到Endpoint
// threadPoolName为线程池的名称,executor为线程池实例
threadPoolEndpoint.registerThreadPool("threadPoolName", executor);
// 可以根据需要注册更多的线程池
}
}
```
启动Spring Boot项目后,可以使用JMX工具来查看并管理线程池信息。可以通过Jvisualvm、JConsole等工具来连接应用程序,查看`com.example.actuator`目录下的`threadpools`节点来获取全部线程池信息。
### 回答3:
要查看Spring Boot项目中的所有线程池信息,可以使用Java Management Extensions(JMX)来实现。下面是具体的步骤:
1. 在Spring Boot项目中添加相关依赖。可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
这些依赖将启用Spring Boot的Actuator功能和web功能。
2. 配置JMX相关设置。在application.properties文件中添加以下配置:
```
# 启用Spring Boot Actuator Endpoints
management.endpoints.jmx.exposure.include=*
# 开启JMX
spring.jmx.enabled=true
spring.main.allow-bean-definition-overriding=true
```
3. 编写一个简单的Controller类用于获取线程池信息。在这个类中,你可以使用Java的ManagementFactory类来获取所有线程池的名称,并通过JMX获取具体信息。下面是一个示例代码:
```java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.management.MBeanServer;
import javax.management.ObjectName;
import java.lang.management.ManagementFactory;
import java.util.ArrayList;
import java.util.List;
@RestController
public class ThreadPoolController {
@GetMapping("/threadPoolInfo")
public List<String> getThreadPoolInfo() throws Exception {
MBeanServer server = ManagementFactory.getPlatformMBeanServer();
ObjectName name = new ObjectName("java.util.concurrent:type=Executor,*");
List<String> threadPoolInfo = new ArrayList<>();
for (ObjectName objectName : server.queryNames(name, null)) {
String poolName = objectName.getKeyProperty("name");
threadPoolInfo.add(poolName);
// 可以根据需要获取更多线程池的具体信息,如线程数、活跃线程数等
// 例如:int poolSize = (int) server.getAttribute(objectName, "PoolSize");
}
return threadPoolInfo;
}
}
```
4. 启动Spring Boot应用程序并访问`http://localhost:8080/threadPoolInfo`,将返回所有线程池的名称列表。如果你需要更多线程池的详细信息,可以根据需要获取。
通过以上步骤,你就可以在Spring Boot项目中查看所有线程池的信息了。
阅读全文
相关推荐
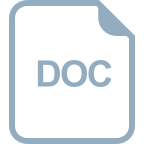
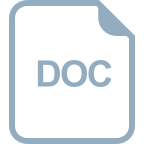



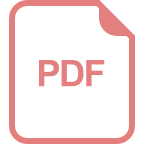











