js 查找一个字符串数组中关键字出现的所有位置
时间: 2024-09-22 13:02:33 浏览: 33
在JavaScript中,你可以通过循环遍历数组并使用`indexOf()`函数或更现代的`Array.prototype.includes()`来查找一个关键词在字符串数组中出现的位置。这里是一个简单的示例:
```javascript
function findKeywordPositions(array, keyword) {
let positions = [];
for (let i = 0; i < array.length; i++) {
if (array[i].includes(keyword)) {
positions.push(i);
}
}
return positions;
}
// 示例
const stringArray = ['apple', 'banana', 'cherry', 'banana'];
const keyword = 'banana';
const result = findKeywordPositions(stringArray, keyword);
console.log(`Keyword "${keyword}" found at positions:`, result);
相关问题
js 查找一个数组中关键字出现的所有位置
在JavaScript中,你可以使用`Array.prototype.map()`、`Array.prototype.filter()`和`indexOf()`或`includes()`方法结合来查找数组中关键字出现的所有位置。以下是一个简单的示例:
```javascript
function findPositions(arr, keyword) {
return arr.map((value, index) => value === keyword ? index : null).filter(index => index !== null);
}
// 示例
const numbers = [1, 2, 3, 4, 5, 2, 6, 2];
const keyword = 2;
const positions = findPositions(numbers, keyword);
console.log(positions); // 输出:[1, 3, 5, 7]
```
这个函数首先创建了一个新数组,其中每个元素都是原数组对应位置的值是否等于关键字。然后,它过滤出结果数组中非`null`的元素(即关键词存在的位置)。如果你需要处理字符串或其他类型的数组,只需要改变比较条件即可。
js 判断数组中的json是否包含指定字符串
可以使用 JavaScript 的 `filter` 方法来判断数组中的 JSON 是否包含指定字符串。具体实现可以参考以下代码:
```javascript
const arr = [
{ name: 'John', age: 25 },
{ name: 'Alice', age: 30 },
{ name: 'Bob', age: 35 }
];
const keyword = 'Alice';
const result = arr.filter(item => {
return JSON.stringify(item).indexOf(keyword) !== -1;
});
console.log(result); // [{ name: 'Alice', age: 30 }]
```
在上面的代码中,我们首先定义了一个包含多个 JSON 的数组 `arr`,然后定义了一个关键字 `keyword`,表示要查找的字符串。接着我们使用 `filter` 方法对数组中的每个 JSON 进行过滤,通过 `JSON.stringify(item)` 将 JSON 转换为字符串,并使用 `indexOf` 方法查找关键字是否存在于字符串中,如果存在,则返回 `true`,否则返回 `false`。最终得到的结果是一个新的数组,其中包含了所有符合条件的 JSON。
阅读全文
相关推荐
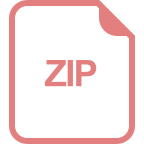
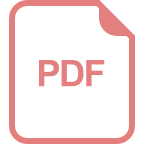
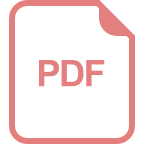
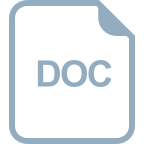
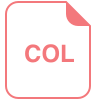
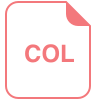
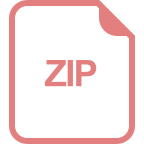
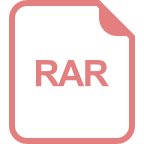
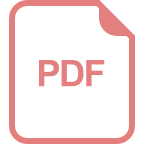
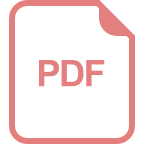
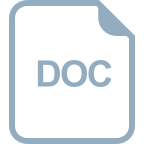
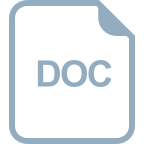
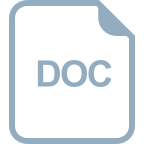
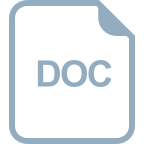
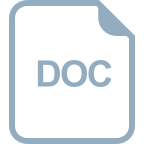
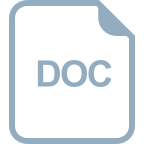
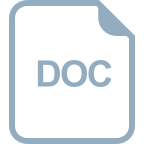