使用torch定义一个包含自编码器损失和自对抗负采样损失函数的模型,(自对抗负采样损失采用RotatE中的负采样策略)并给出实例看如何使用模型
时间: 2024-02-21 09:56:54 浏览: 113
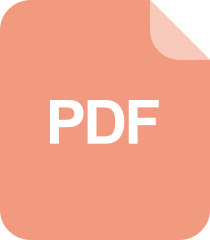
Pytorch 的损失函数Loss function使用详解
好的,我来为你解答。
首先,我们需要导入必要的库:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.autograd import Variable
```
然后,我们定义模型。这里以一个简单的自编码器为例:
```python
class AutoEncoder(nn.Module):
def __init__(self, input_dim, hidden_dim):
super(AutoEncoder, self).__init__()
self.encoder = nn.Linear(input_dim, hidden_dim)
self.decoder = nn.Linear(hidden_dim, input_dim)
def forward(self, x):
encoded = F.relu(self.encoder(x))
decoded = F.relu(self.decoder(encoded))
return decoded
```
接着,我们定义自对抗负采样损失函数。这里以RotatE中的负采样策略为例:
```python
class NegativeSampling(nn.Module):
def __init__(self, num_entities, num_relations, embedding_dim, margin=1.0, corruption_rate=0.5):
super(NegativeSampling, self).__init__()
self.num_entities = num_entities
self.num_relations = num_relations
self.embedding_dim = embedding_dim
self.margin = margin
self.corruption_rate = corruption_rate
self.entity_embeddings = nn.Embedding(num_entities, embedding_dim)
self.relation_embeddings = nn.Embedding(num_relations, embedding_dim)
self.entity_embeddings.weight.data.uniform_(-1, 1)
self.relation_embeddings.weight.data.uniform_(-1, 1)
def forward(self, positive_samples):
batch_size, num_sampled = positive_samples.shape[0], 1
negative_samples = torch.randint(low=0, high=self.num_entities, size=(batch_size, num_sampled)).to(positive_samples.device)
corrupted_samples = torch.where(torch.rand(batch_size, self.embedding_dim).to(positive_samples.device) < self.corruption_rate, negative_samples, positive_samples)
positive_scores = self._calc_score(positive_samples)
negative_scores = self._calc_score(corrupted_samples)
loss = F.relu(self.margin - positive_scores + negative_scores).mean()
return loss
def _calc_score(self, samples):
head, relation, tail = torch.chunk(samples, chunks=3, dim=1)
head_emb = self.entity_embeddings(head).view(-1, self.embedding_dim)
relation_emb = self.relation_embeddings(relation).view(-1, self.embedding_dim)
tail_emb = self.entity_embeddings(tail).view(-1, self.embedding_dim)
score = torch.sum(head_emb * relation_emb * tail_emb, dim=1)
return score
```
最后,我们将两个损失函数结合起来,定义整个模型:
```python
class Model(nn.Module):
def __init__(self, input_dim, hidden_dim, num_entities, num_relations, embedding_dim, margin=1.0, corruption_rate=0.5):
super(Model, self).__init__()
self.autoencoder = AutoEncoder(input_dim, hidden_dim)
self.negsampling = NegativeSampling(num_entities, num_relations, embedding_dim, margin, corruption_rate)
def forward(self, x, positive_samples):
reconstructed = self.autoencoder(x)
loss_ae = F.mse_loss(reconstructed, x)
loss_ns = self.negsampling(positive_samples)
return loss_ae, loss_ns
```
其中,`input_dim`表示自编码器的输入维度,`hidden_dim`表示自编码器的隐藏层维度,`num_entities`表示实体总数,`num_relations`表示关系总数,`embedding_dim`表示实体和关系的嵌入维度,`margin`表示自对抗负采样损失函数中的margin,`corruption_rate`表示负采样时,对每个实体的每个维度进行替换的概率。
使用模型时,我们需要提供自编码器的输入`x`和正样本`positive_samples`,并计算出总的损失函数:
```python
model = Model(input_dim=100, hidden_dim=50, num_entities=10000, num_relations=100, embedding_dim=50)
x = torch.randn(32, 100)
positive_samples = torch.randint(low=0, high=10000, size=(32, 3), dtype=torch.long)
loss_ae, loss_ns = model(x, positive_samples)
total_loss = loss_ae + loss_ns
```
以上就是使用torch定义一个包含自编码器损失和自对抗负采样损失函数的模型的示例。
阅读全文
相关推荐
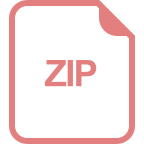
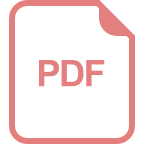
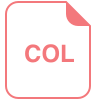
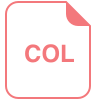
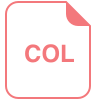
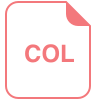
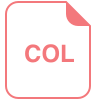
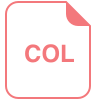
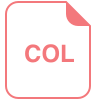
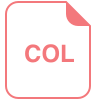
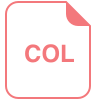
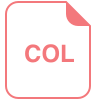
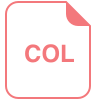
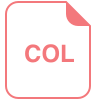
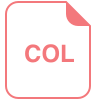
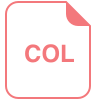
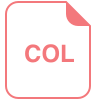
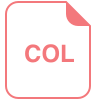