帮我用python、vue写一个websocket远程桌面共享的例子,尽可能详细
时间: 2023-06-07 18:07:42 浏览: 79
好的,我可以为您提供一个基于Python和Vue的WebSocket远程桌面共享的示例。下面是我提供的代码示例:
Python部分:
1. 安装必要的库:
```python
pip install websockets pillow autopy
```
2. 服务器端代码:server.py
```python
import websockets
from PIL import ImageGrab
import autopy
import asyncio
# 获取桌面截图
async def grab_screenshot():
while True:
image = ImageGrab.grab()
yield image.tobytes()
# 处理客户端发送的消息
async def message_handler(websocket, path):
async for message in websocket:
# 如果客户端发送了“move”消息,移动鼠标
if message == "move":
mouse_x, mouse_y = autopy.mouse.get_pos()
autopy.mouse.move(mouse_x + 10, mouse_y + 10)
# 如果客户端发送了“click”消息,模拟鼠标点击
elif message == "click":
autopy.mouse.click()
# 如果客户端发送了“screenshot”消息,发送当前桌面截图
elif message == "screenshot":
async for image_bytes in grab_screenshot():
await websocket.send(image_bytes)
# 启动WebSocket服务器
start_server = websockets.serve(message_handler, 'localhost', 8080)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
```
Vue部分:
1. 创建Vue项目:
```bash
vue create client
```
2. 安装必要的库:
```bash
npm install --save vue-websocket axios
```
3. 编写Vue组件:App.vue
```vue
<template>
<div class="wrapper">
<canvas ref="canvas" v-show="showCanvas"></canvas>
<div class="controls">
<button @click="moveMouse">Move Mouse</button>
<button @click="clickMouse">Click Mouse</button>
<button @click="takeScreenshot">Take Screenshot</button>
</div>
</div>
</template>
<script>
import VueWebSocket from "vue-websocket";
import axios from "axios";
export default {
name: "App",
mixins: [VueWebSocket("ws://localhost:8080/")],
data() {
return {
showCanvas: false,
};
},
mounted() {
// 监听鼠标移动事件
this.$refs.canvas.addEventListener("mousemove", (event) => {
if (this.ws.readyState === WebSocket.OPEN) {
const message = {
type: "move",
x: event.clientX,
y: event.clientY,
};
this.$socket.send(JSON.stringify(message));
}
});
// 监听鼠标点击事件
this.$refs.canvas.addEventListener("click", (event) => {
if (this.ws.readyState === WebSocket.OPEN) {
const message = {
type: "click",
x: event.clientX,
y: event.clientY,
};
this.$socket.send(JSON.stringify(message));
}
});
},
methods: {
// 移动鼠标
moveMouse() {
const message = { type: "move" };
this.$socket.send(JSON.stringify(message));
},
// 模拟鼠标点击
clickMouse() {
const message = { type: "click" };
this.$socket.send(JSON.stringify(message));
},
// 获取桌面截图
async takeScreenshot() {
try {
this.showCanvas = true;
const canvas = this.$refs.canvas;
const ctx = canvas.getContext("2d");
const img = document.createElement("img");
const imageBytes = await axios.get(
"http://localhost:8080/screenshot",
{ responseType: "arraybuffer" }
);
img.src = window.URL.createObjectURL(
new Blob([imageBytes.data], { type: "image/png" })
);
img.onload = () => {
ctx.drawImage(img, 0, 0, canvas.width, canvas.height);
};
} catch (error) {
console.error(error);
} finally {
this.showCanvas = false;
}
},
},
};
</script>
<style>
.wrapper {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
canvas {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
z-index: -1;
}
.controls {
display: flex;
flex-direction: column;
align-items: center;
}
button {
margin: 16px 0;
padding: 8px 16px;
font-size: 16px;
border: none;
border-radius: 4px;
background-color: #f1f1f1;
cursor: pointer;
}
</style>
```
这是一个非常基本的远程桌面共享示例,但它足以让你开始学习和开发WebSocket应用程序。希望对您有所帮助!
相关推荐
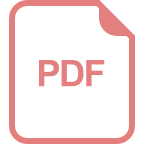
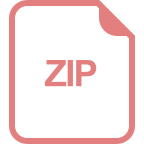














