java实现ftp下载上传
时间: 2023-10-21 14:05:00 浏览: 62
Java可以通过FTPClient类来实现FTP的上传和下载。下面是一个简单的示例代码:
上传文件:
```java
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(server, port);
ftpClient.login(user, password);
ftpClient.enterLocalPassiveMode();
File file = new File(localFilePath);
String remoteFilePath = "/remote/path/file.txt";
InputStream inputStream = new FileInputStream(file);
ftpClient.storeFile(remoteFilePath, inputStream);
inputStream.close();
} catch (IOException ex) {
ex.printStackTrace();
} finally {
try {
if (ftpClient.isConnected()) {
ftpClient.logout();
ftpClient.disconnect();
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
```
下载文件:
```java
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(server, port);
ftpClient.login(user, password);
ftpClient.enterLocalPassiveMode();
String remoteFilePath = "/remote/path/file.txt";
File downloadFile = new File(localFilePath);
OutputStream outputStream = new BufferedOutputStream(new FileOutputStream(downloadFile));
ftpClient.retrieveFile(remoteFilePath, outputStream);
outputStream.close();
} catch (IOException ex) {
ex.printStackTrace();
} finally {
try {
if (ftpClient.isConnected()) {
ftpClient.logout();
ftpClient.disconnect();
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
```
相关推荐
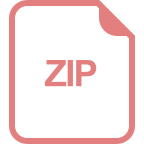
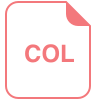
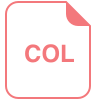
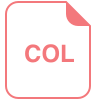
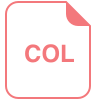










